How to Rotate an Object Programmatically in Fabric JS
Fine tune your canvas app by learning how to rotate an object programmatically in Fabric JS.
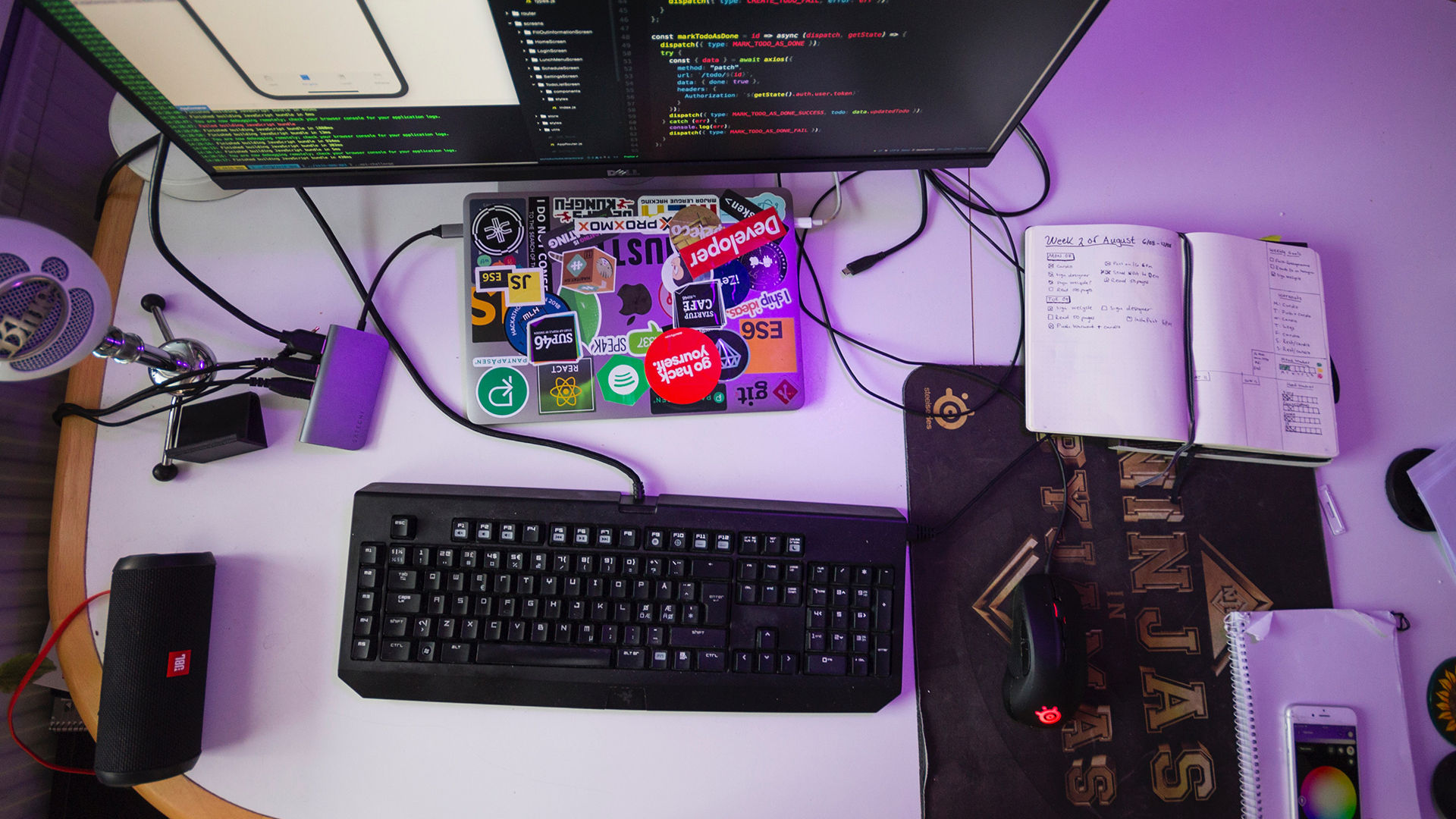
There are many default ways to manipulate objects in Fabric JS. When making a selection, you are given the ability to move, resize and rotate an object using the corner controls. But sometimes, you need more refined control, specifically in the degrees of rotation. Here is how to rotate an object programmatically in Fabric JS.
How to Rotate an Object Programmatically in Fabric JS
To rotate an object programmatically in Fabric JS, you must call the rotate()
method on the object.
let activeObj = canvas.getActiveObject()
//Increment the angle by 10 degrees
let angle = activeObj.angle
let newAngle = angle == 355 ? 0 : angle + 10
activeObj.rotate(newAngle) //Rotate Here
canvas.renderAll()
The rotation of an object is performed by the rotate() method in Fabric JS. To modify this property, you must first retrieve your object. If the object you want to modify is currently selected on the canvas, you can call canvas.getActiveObject(); otherwise, you will want to save and retrieve the object somewhere in your code.
Typically if you want to rotate an object programmatically, it’s because you want to rotate it in increments. You can grab the object’s current angle by calling the angle property to achieve this. With this angle, you can add the number of degrees for your rotation increment and call rotate() to perform the rotation.
Don’t forget to perform canvas.renderAll()
to rerender the canvas after calling an object rotation.
Disable Center Rotation in Fabric JS
When an object is created in Fabric JS, it rotates around its center by default. This is often the desired rotation point, but you may have a specific use case where you need your object to rotate around the edge. This is more common when using an Image object. To achieve this, you must set the centeredRotation
property to false on your object.
let obj = new fabric.Rect({
width: 500,
height: 200,
fill: 'red',
centeredRotation: false
});
canvas.add(obj)
Related: How to Add Custom Params to a Fabric JS Object
This property can be set during initialization but can also be changed anytime by using the set()
method on your object.
You now have the basic groundwork for rotating an object programmatically in Fabric JS. Hopefully, this new set of methods will take your app to the next level. Happy coding!
Helpful References:
For more useful Fabric JS tips, tricks, and guides, check out our Fabric JS section.