How to Add Custom Params to a Fabric JS Object
Learn how to add custom params to a Fabric JS Object with these simple code snippets.
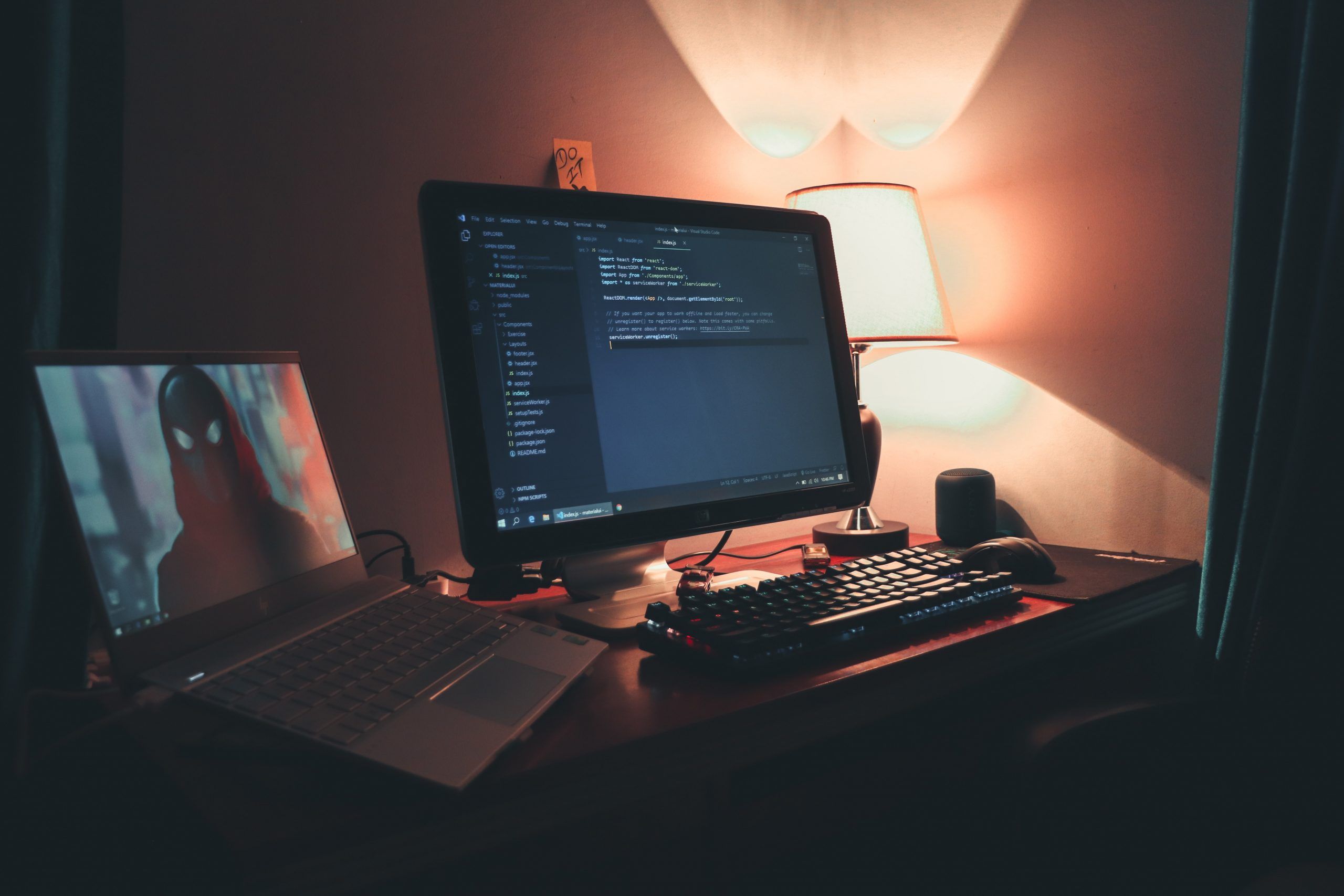
Fabric JS is a powerful canvas manipulation library with many helpful built-in functions to build a highly customizable app. If you need to extend the base parameters of a regular Fabric JS object, look no further. Here is how to add custom parameters to a Fabric JS Object.
How to Add Custom Params to a Fabric JS Object
To add custom parameters (or properties) to your Fabric JS object, add your params to the initial object when initializing.
let myObject = new fabric.Rect({
fill: 'red',
width: 200,
height: 200,
specialParam: 'something here'
})
canvas.add(myObject)
Fabric JS objects, whether an image, shape, text or anything else, are JavaScript objects with some base parameters and functions in them. You can add any extra data you like to this object and retrieve it later.
How to Add Custom Params to an Existing Fabric JS Object
If your object was already initialized and you need to add additional parameters to it, you can use the set()
method:
let myObject = new fabric.Rect({
fill: 'red',
width: 200,
height: 200
})
myObject.set({
specialParam: 'something here'
})
canvas.add(myObject)
The set()
method can also be used to add or modify the base parameters, such as width
and height
.
How to Add Dynamic Params to a Fabric JS Object
If you are adding dynamic parameters, you can add them all as an object into a Fabric JS property:
let someDynamicData = {
key1 = 'data1'
key2 = 'can be anything'
}
let myObject = new fabric.Rect({
fill: 'red',
width: 200,
height: 200,
customParams: someDynamicData
})
canvas.add(myObject)
This approach will let you add any objects to your single custom parameter and retrieve the entire object later on in your code:
myObject.get('customParams')
Adding all of your custom parameters into a single variable is a good approach if you will have a lot of custom data or your keys might be dynamic.
How to Retrieve Custom Params to an Existing Fabric JS Object
To retrieve custom params from your Fabric JS object, use the get()
method with your parameter key:
let myObject = new fabric.Rect({
fill: 'red',
width: 200,
height: 200,
specialParam: 'something here'
})
canvas.add(myObject)
let paramValue = myObject.get('specialParam')
The get()
method is a built-in Fabric JS function that will parse your object and retrieve the data set in the key.
You should now have a solid grasp on how to add custom params to a Fabric JS object. Without the techniques above, you may have to create a secondary lookup object to retrieve customer information making your code needlessly complex.
Check out our Javascript coding section for more useful tips, tricks, and best practices for your JS project.