How to Perform String Interpolation in JavaScript
Learn how to embed variables directly into strings using string interpolation in JavaScript.
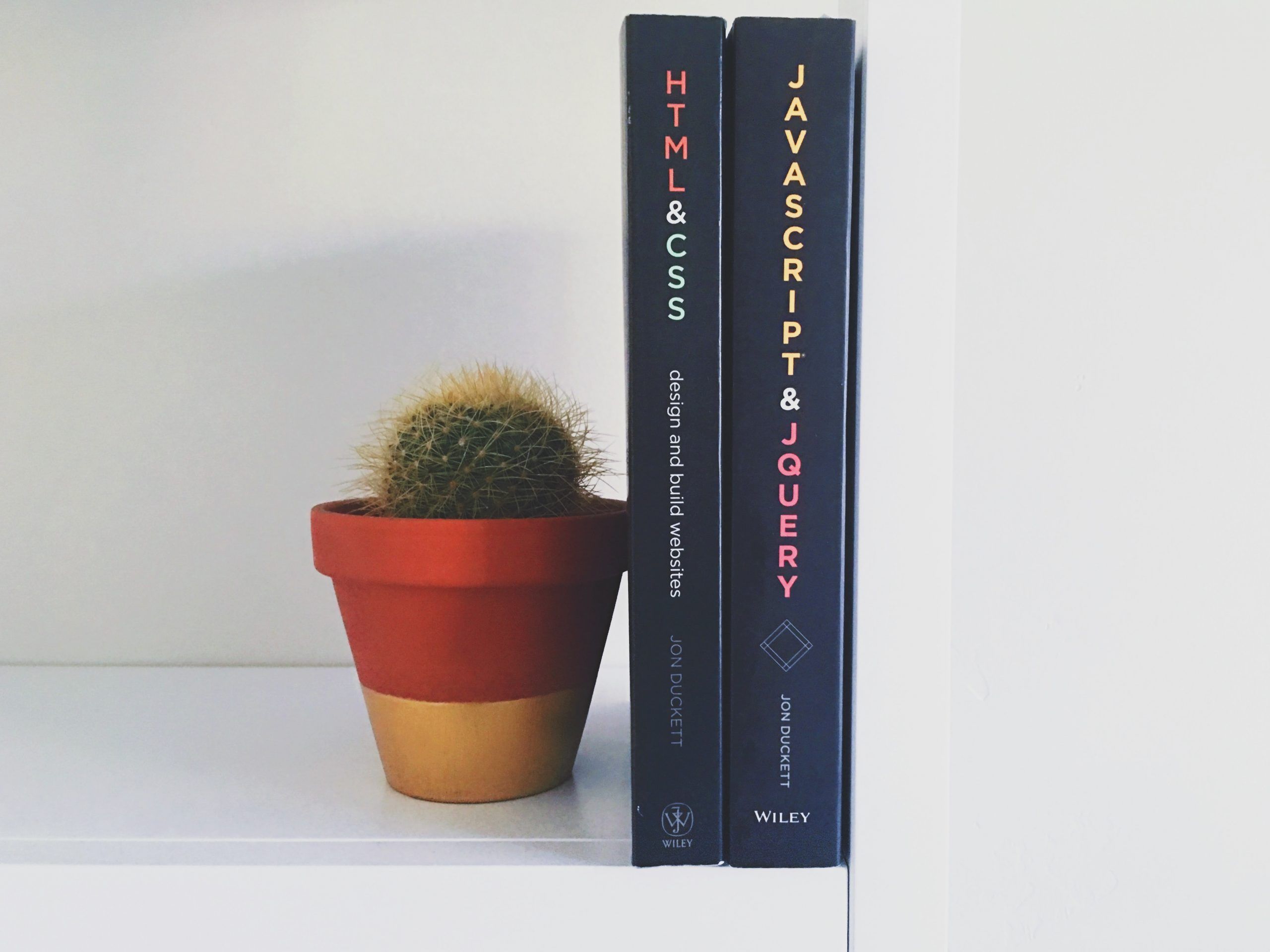
To perform string interpolation in Javascript, you must wrap your string with backticks and then your variable with brackets and prepend a dollar sign. Here is an example:
let name = 'Techozu';
let tagline = `Welcome to ${name}!`;
console.log(tagline)
// OUTPUT
// Welcome to Techozu!
In this example, our string is wrapped with backticks `
and our variable name
is wrapped with curly brackets {}
. Prepending the $
key will evaluate our placeholder within the string and give the desired response.
Why Use String Interpolation?
If you want to display a javascript variable directly inside a string, then you’re looking to perform a string interpolation. String interpolation is a technique used to create cleaner, more readable markup. It’s a good rule to consider string interpolation when you find yourself performing direct string concatenation. Let’s look at an example:
let customer = 'Bob'
let vehicle = 'Jeep Renegade'
let date = 'October 2nd'
let output = 'Our customer ' + customer + ', purchased his ' + vehicle + ' on October 2nd.'
console.log(output)
// OUTPUT
// Our customer Bob, purchased his Jeep Renegade on October 2nd.
The cleaned-up string interpolated version would look like this:
let customer = 'Bob'
let vehicle = 'Jeep Renegade'
let date = 'October 2nd'
let output = `Our customer ${customer}, purchased his ${vehicle} on ${date}`
console.log(output)
// OUTPUT
// Our customer Bob, purchased his Jeep Renegade on October 2nd.
The modified code is shorter and much easier to read at a glance.
Your placeholders aren’t limited to just plain string variables. They also execute regular javascript, meaning you can write more complex JavaScript code into them. Let’s take a look at an example:
let colors = ['red','green','blue']
//Output random color
let output = `The color is ${colors[Math.floor(Math.random() * colors.length)]}`
console.log(output)
// OUTPUT
// The color is blue
It’s not best practice to perform complex JavaScript logic directly inside your strings. Move logic into separate variables to make your code more robust.
The Different String in Javascript
Strings in JavaScript can be wrapped in one of three ways:
‘ Single Quote
let output = 'Hello from Techozu'
“ Double Quote
let output = "Hello from Techozu"
` Tilde (backtick)
let output = `Hello from Techozu`
Which one you use will mostly depend on what your string is. If your string is an HTML snippet, you may want to use single quotes. If you have a lot of single quotes in your string, you may want to wrap it in double quotes. If you need to perform string interpolation with a placeholder, you must use backticks.
How to Escape Strings in Javascript
Strings can be escaped in Javascript using the backslash \
key. You can even escape your placeholder using it, like so:
let name = 'Techozu';
let tagline = `Use a placeholder like this \${name}`;
console.log(tagline)
// OUTPUT
// Use a placeholder like this ${name}
Other times escaping strings will be necessary is when your string wrapping character is inside the string itself. For example, if you wrapped your string with single quotes but your string also contains single quotes.
let tagline = 'Single quotes \' need to be escaped here';
console.log(tagline)
// OUTPUT
// Single quotes ' need to be escaped here
That’s all there is to embedding variables into your strings using JavaScript string interpolation. Use this simple pattern to make your code better.
For helpful guides, tips, tricks, and more, head to our JavaScript Coding Section.