How to Split an Array into Chunks in JavaScript
Need to learn how to split an array into chunks? We got you covered with this simple JavaScript solution.
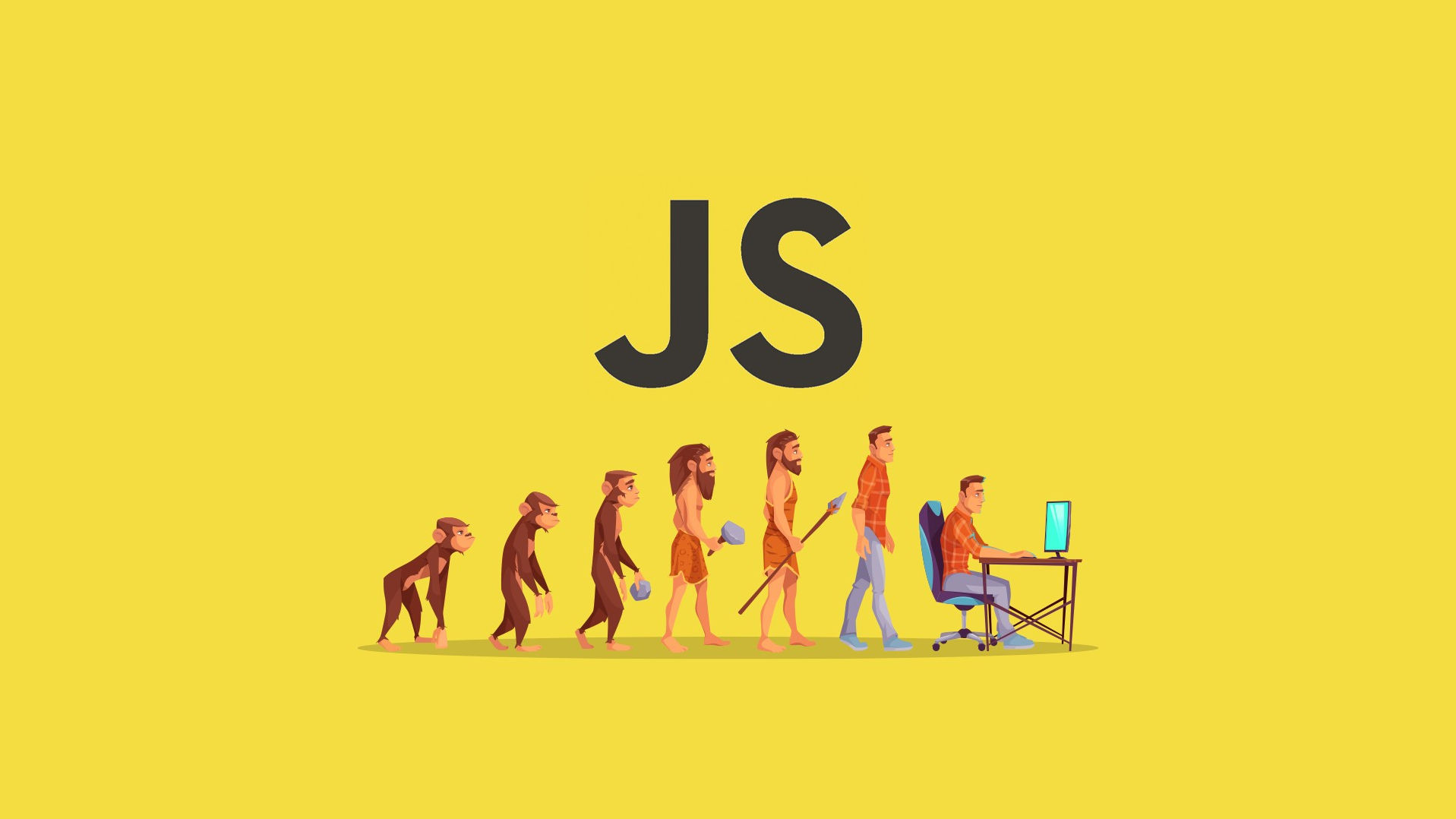
When working with arrays, you may need to perform tons of unique operations on them. One such operation is splitting an array into chunks—meaning taking an array of elements and breaking it into sub-arrays of predefined length. While there are many solutions to this problem, we will share our favorite. Here is how to chunk a javascript array.
How to Split an Array into Chunks in JavaScript (Solution)
You can use the slice()
method within a loop to split an array into chunks. Let’s take a look at a code example:
const input_array = ['a','b','c','d','e','f']
const chunk_size = 2;
let output_array = []
for (let i = 0; i < input_array.length; i += chunk_size) {
const chunk = input_array.slice(i, i + chunk_size);
output_array.push(chunk)
// Your chunk operations here
}
console.log(output_array)
// OUTPUT
// [["a", "b"], ["c", "d"], ["e", "f"]]
This method uses a simple for-loop
, with the limit being the input array size. The loop is incremented by the chunk size. Within the loop, we slice
our array from the current loop index up to the index, plus our chunk size. Inside the loop, you can perform any action you like, but in our example, we pushed the chunk into a new array of chunks. You could perform different actions, such as async operations on your chunk array at that point.
Careful that chunk_size
is not set to zero; this can cause an infinite loop
This particular code example assumes that we have a static chunk size set, but if your chunk size is dynamic, you will want to assert that it isn’t zero, so you don’t enter an infinite loop. Here is a simple example that checks the chunk size.
const input_array = ['a','b','c','d','e','f']
const chunk_size = 2;
let output_array = []
if (chunk_size < 1) throw 'Chunk Size Cannot be Zero' //OR return
for (let i = 0; i < input_array.length; i += chunk_size) {
const chunk = input_array.slice(i, i + chunk_size);
output_array.push(chunk)
// Your chunk operations here
}
This example throws or returns an error if the chunk size is zero before entering into the loop.
The solution above is just one of the many methods you can use to split an array into chunks. Its advantages are that the code is straightforward and readable, making it easy to understand when you return to your code after a long hiatus or when collaborating. In addition, this method is also relatively efficient. You can write this method directly into an array prototype so it can be reused easily.
Object.defineProperty(Array.prototype, 'chunk', {
value: function(chunk_size) {
let output_array = [];
for (let i = 0; i < this.length; i += chunk_size) {
const chunk = this.slice(i, i + chunk_size);
output_array.push(chunk);
}
return output_array
}
})
const input_array = ['a','b','c','d','e','f']
let chunked_array = input_array.chunk(2)
console.log(chunked_array)
// OUTPUT
// [["a", "b"], ["c", "d"], ["e", "f"]]
Of course, if you know you will only be using this once in your codebase, it may be unnecessary to define a prototype.
Now you know how to split an array into chunks in JavaScript.
We hope you found this guide helpful. For more coding help, please check out our Javascript coding section.