How to Sleep in JavaScript
Delay your code execution by learning how to sleep in JavaScript. Prevent hitting API rate limits and more.
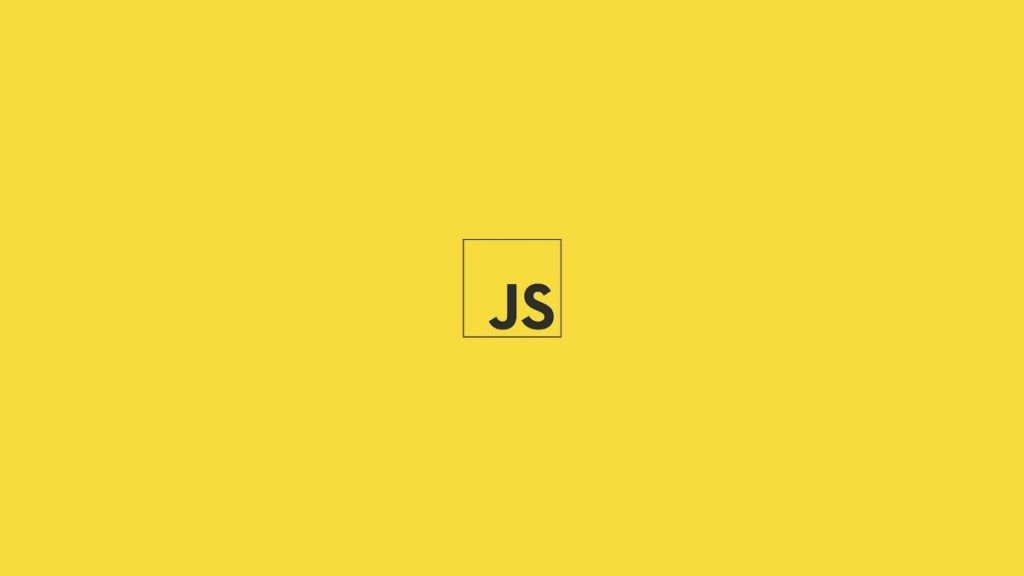
Sleep functions are a must for any programming language. There are many use cases where you need to delay a function’s execution. One example would be the need to create a delay between multiple API calls in order not to hit rate limits. Whatever your needs are, here is how to sleep in JavaScript.
How to Sleep in JavaScript (Solution)
To perform a sleep in JavaScript, we must write a Promise
that resolves a timeout function. Let’s take a look at the code:
const sleep = ms => new Promise(resolve => setTimeout(resolve, ms));
The shorthand for this code is simple. We are creating a sleep function that takes in our sleep time in milliseconds and returns a Promise
. The promise will resolve after a timeout which is started with setTimeout()
function.
The code above writes our function to a variable, but you can also write it as a regular function:
function sleep(ms) {
return new Promise(resolve => setTimeout(resolve, ms));
}
Both of the above solutions accomplish the same thing, with different syntax arrangements.
Now we just have to use our new sleep function. We can await
our sleep function inside any async
function. Let’s take a look.
const sleep = ms => new Promise(resolve => setTimeout(resolve, ms));
async function callAPI() {
console.log('Hello')
await sleep(2000)
console.log('from Techozu')
}
// OUTPUT
// Hello
// **2 second delay**
// from Techozu
This solution, with its usage of async/await
, will work with Node 7+ and Chrome 55+
If you prefer to use seconds instead of milliseconds, you can either multiple your input by 1000 or multiply the setTimeout duration by 1000.
await sleep(2 * 1000) //2 second delay
// OR
const sleep = s => new Promise(resolve => setTimeout(resolve, s * 1000));
You can use the then
chain if you plan to use your new sleep method without an async function.
sleep(2000).then(() => { console.log('Hello from Techozu')}
// OUTPUT
// **2 second delay**
// Hello from Techozu
This, however, is only applicable for specific scenarios. You always want to avoid excessively nested code, which will significantly reduce code readability.
Now you have a simple function to sleep in JavaScript. You can always pull this code into a reusable utility class that you can use across multiple projects.
For more helpful Javascript tips and tricks, check out our Javascript and Node Coding Section.