How to Shuffle an Array in Javascript
Use these two methods to shuffle an array in Javascript.
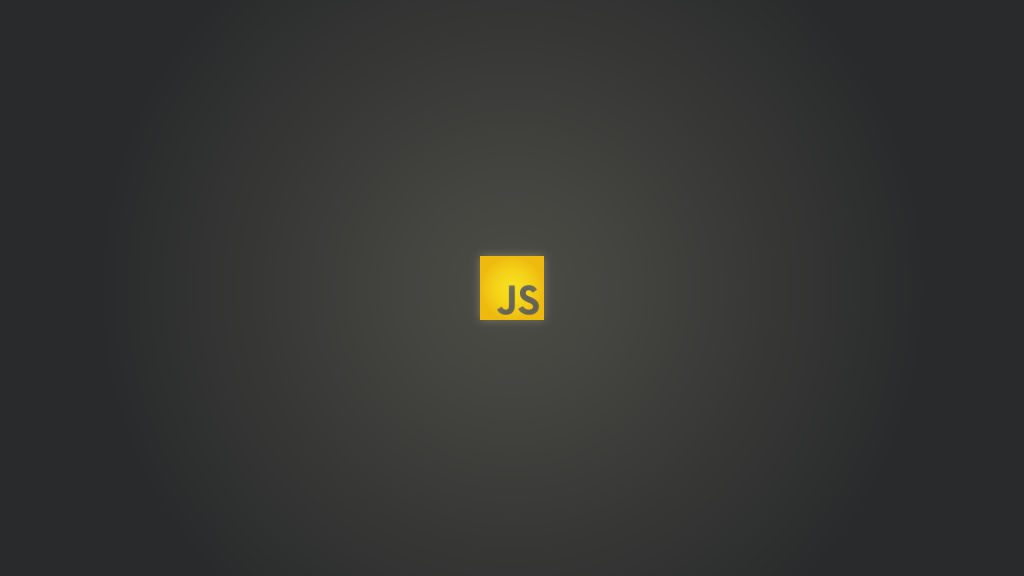
There are many ways to shuffle an array in javascript, and your shuffling criteria should always fall under efficiency and randomness when choosing a solution. Here we will provide you with two solutions, the Fisher-Yates method and the sort one-liner. Both solutions come with their own advantages and disadvantages, so it will be up to you to decide which method fits best with your project. Here is how to shuffle an array in Javascript.
Solution 1: Fisher-Yates Array Shuffle
let array = ['a','b','c','d','e']
function FisherShuffle(temp) {
let i = temp.length;
while (--i > 0) {
let randIndex = Math.floor(Math.random() * (i + 1));
[temp[randIndex], temp[i]] = [temp[i], temp[randIndex]];
}
return temp;
}
A popular array shuffling solution in Javascript is the Fisher-Yates shuffle. The concept here is simple:
- Start at the end of your array.
- Choose a random index within your array
- Swap the two values
- Repeat down your array until all indexes are swapped.
This method will provide you with great randomness, and although it’s relatively efficient, its performance will be impacted by the size of the array. Because we have to write a bit of code to perform the shuffle, it’s best practice to put the algorithm in a reusable function, preferably in a utility file you can call in different parts of your project.
The Fisher-Yates shuffle was first described in 1938 and is named after its creators, Ronald Fisher and Frank Yates. It is also sometimes referred to as the Knuth shuffle.
Solution 2: Sort One Liner
let array = ['a','b','c','d','e']
let shuffled = array.sort( () => .5 - Math.random() )
Many people love this solution for its cleanness and simplicity. However, it has some significant drawbacks. It isn’t very efficient for large arrays, and many elements can be left unshuffled, leaving you with poor overall randomness. It would be best if you only used this solution on small arrays where high percentage randomness isn’t critical.
Those are just two of the solutions for how to shuffle an array in Javascript. We highly recommend using the first Fisher-Yates method from these two options, even though it has a bigger syntax payload. Hopefully, you found this guide useful. For more useful programming tutorials, head over to our javascript section.