How to Show All Items When You console.log in Node
Avoid the more items message and show all items when you console log in Node.
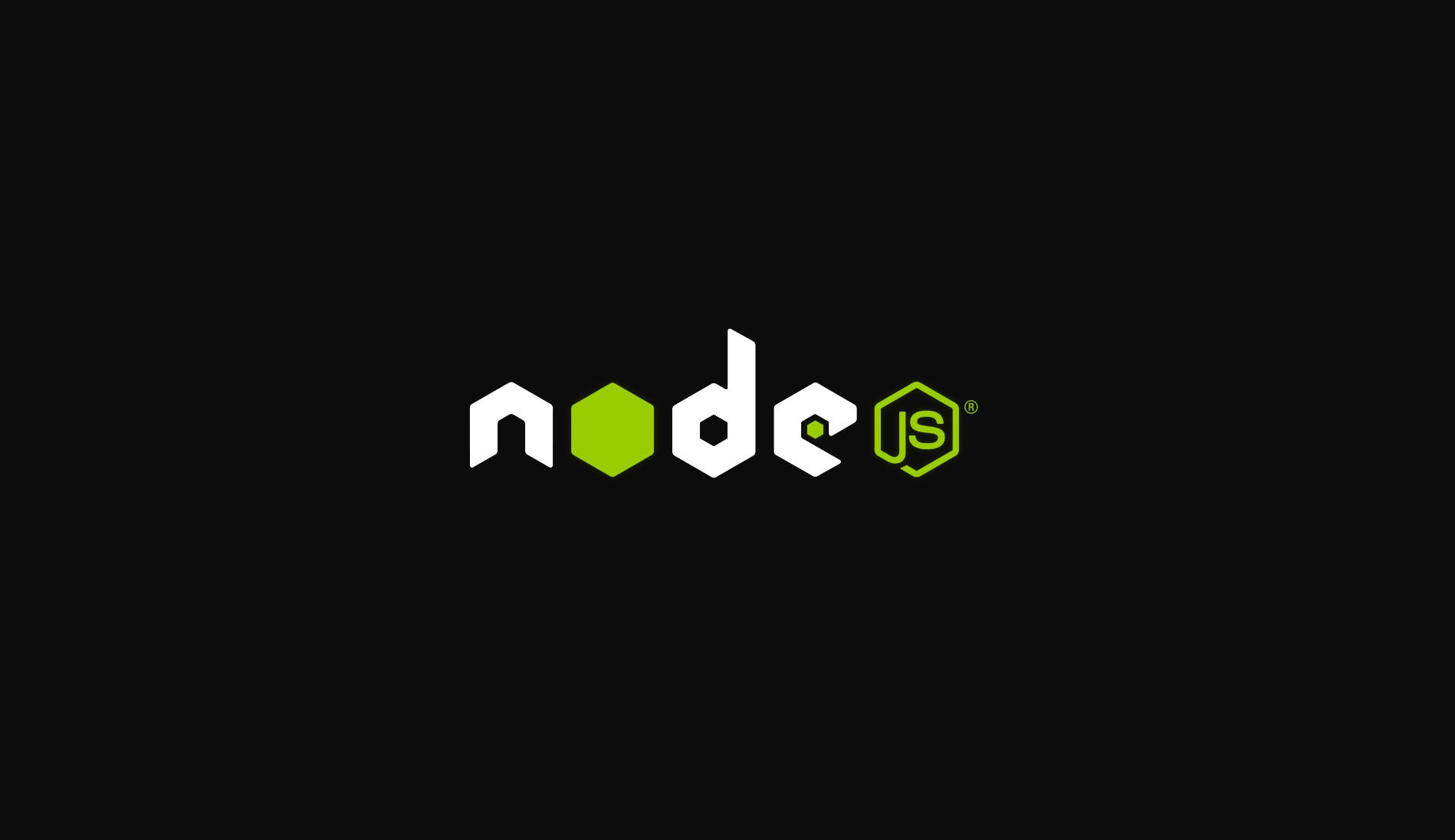
While working on any NodeJS project, you will perform tons of console logs, either debugging or extracting information from your code. If you work with large arrays, you may notice odd behavior from the console.log() command. Your terminal will not display all values with large arrays or objects. Instead, it will display a few values and then say “more items” to signify that your data structure still has more items that can potentially be shown. Here is how to show all items when you console.log in Node.
//Country console.log Example
[
'United States',
'Canada',
'Mexico',
'Brazil',
'China',
'Japan',
... 189 more items
]
How to Show All Items When You console.log in Node JS
To show all array items when you console.log to your terminal in Node JS. Use the console.dir() method.
let countries = require('./countries.json')
console.dir(countries, {'maxArrayLength': null})
// This provides a full array output
Console.log isn’t the only way to log data in javascript. You also have a few other options, one of which is console.dir(). The console.dir() method is very useful when you want to display objects, especially in a hierarchical structure. This method will allow you to take an array and force the console to show all its items. In the code example, you input your array as you usually would, but you also add an extra object parameter with the key-value pair of ‘maxArrayLength’: null.
Another option is to use the console.table() method.
let countries = require('./countries.json')
console.table(countries)
//OUTPUT
/**
┌─────────┬────────────────────────────────────────────────────────┐
│ (index) │ Values │
├─────────┼────────────────────────────────────────────────────────┤
│ 0 │ 'United State' │
│ 1 │ 'Canada' │
│ 2 │ 'Mexico' │
│ 3 │ 'Brazil' │
│ 4 │ 'Angola' │
│ 5 │ 'Antigua and Barbuda' │
│ 6 │ 'Argentina' │
This table will continue to display your full list
**/
The console.table() method will take an object and format it in a table with multiple columns if you specify more than one. While this approach may not be applicable in some use cases, it is handy if you want a clean and readable output. Although you shouldn’t be using unsupported node versions in your projects, you will need to have Node version 10 or higher to use this method.
If you run into the “…more items” issue in the future, you know now how to show all items when trying to console log in Node js. Happy coding!
We hope you found this guide helpful. For more useful guides, please check out our Javascript coding section.