How to Set the Viewport in Puppeteer
Learn how to set the viewport in your Puppeteer browser page or even emulate an entire device.
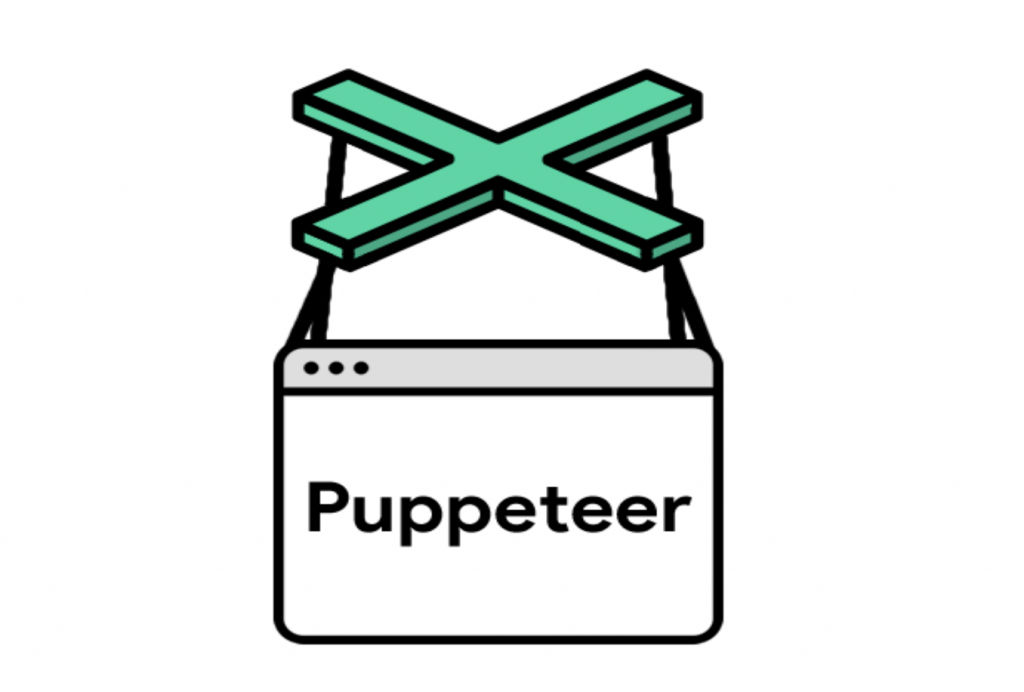
Puppeteer is one of the most popular headless chrome APIs for Node. It comes packed with tons of features and provides simple syntax to work with. Sometimes it’s necessary to change your headless browser’s windows resolution or emulate a specific device. Here is how to set the viewport in Puppeteer.
How to Set the Viewport in Puppeteer
To set the viewport in Puppeteer, use the “setViewport” function on your page object.
const puppeteer = require('puppeteer');
(async () => {
const browser = await puppeteer.launch();
const page = await browser.newPage();
//Code to Set Viewport
await page.setViewport({
width: 1920,
height: 1080
})
await page.goto('https://www.example.com');
await browser.close();
})();
Once you create a new page in your Puppeteer browser instance, you can change its viewport to adjust the resolution. Setting your viewport before navigating to the URL is a good idea. Many sites won’t format correctly if you change the viewport size after loading the page.
The setViewport function will accept an object with the following properties:
- width: pixel width of viewport (required)
- height: pixel height of viewport (required)
- isLandScape: identifies that the viewport is in landscape mode (optional) (default false)
- isMobile: identifies that the viewport is on mobile device (optional) (default false)
- hasTouch: identifies if the viewports supports touch events (optional) (default false)
- deviceScaleFactor: sets the DPR (optional) (default 1)
You must include the width and height when setting the viewport, but the rest of the arguments are optional. Width and height are both set in pixels. Here are some of the most popular resolutions across multiple devices:
Desktop
- 1920×1080
- 1366×768
- 1440×900
- 1280×720
Mobile
- 414×896
- 375×667
- 360×640
- 360×780
Tablet
- 1280×800
- 962×601
- 800×1280
- 768×1024
If you are trying to make your puppeteer instance look like a mobile device, you can use the “emulate” function.
const puppeteer = require('puppeteer');
const iPhone = puppeteer.devices['Galaxy Note II'];
(async () => {
const browser = await puppeteer.launch();
const page = await browser.newPage();
await page.emulate(iPhone);
await page.goto('https://www.example.com');
await browser.close();
})();
The emulate function sets both the user agent and viewport for the specified device. You can get a list of the usable devices along with their resolutions and user agents here.
Now you know how to set the viewport in Puppeteer or directly emulate specific devices.
If you would like to check out more helpful tutorials, check out our Javascript section. Happy Coding!