How to Screenshot an Element in Puppeteer
Create more customized screenshots by learning how to screenshot elements in Puppeteer.
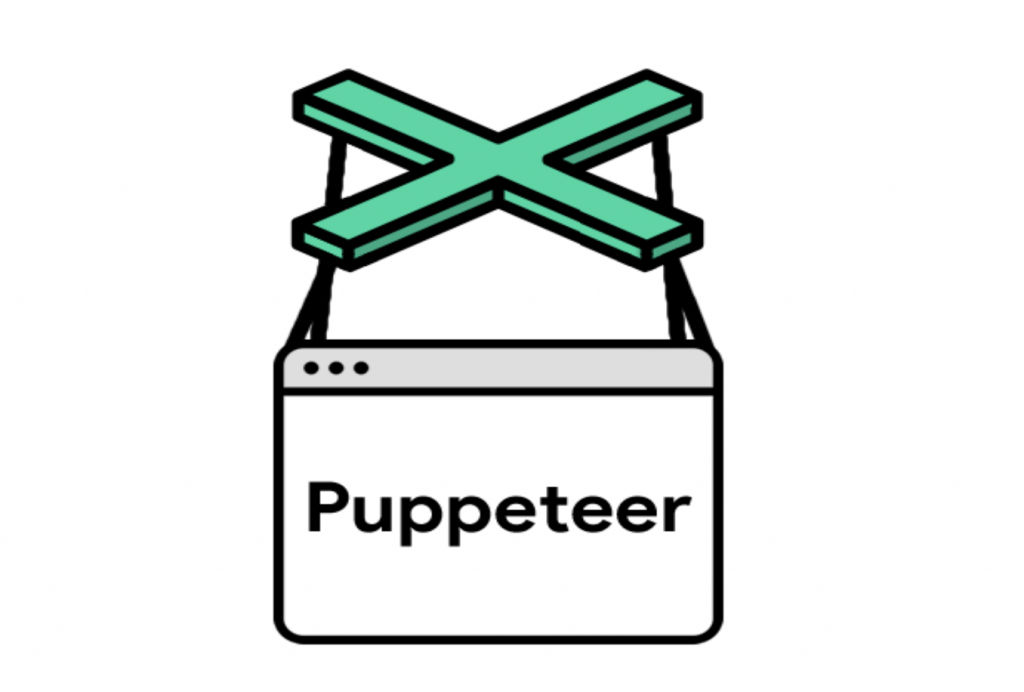
One of the most valuable features of Puppeteer is the ability to take a screenshot of the page and save it as a file. You can use this feature to debug your code or make it directly part of your app. Sometimes it’s useful to generate images of individual elements. Here is how to screenshot an element in Puppeteer.
How to Screenshot an Element in Puppeteer
To screenshot an element in Puppeteer, you must run the screenshot method on your element selector. Let’s take a look at a simple example:
let element = await page.$('.my-element')
await element.screenshot({
path: './tmp/screenshot.png',
})
First, we use the page.$()
method to get our element reference, with your selector as the parameter. This method will run document.querySelector
inside of the page. Once we have this reference, we simply call the screenshot()
method to create an image of just the element alone.
Page.$()
calls document.querySelector while Page.$$()
calls document.querySelectorAll and returns an array.
The `screenshot method takes a path parameter which is a relative path to the current working directory. If you omit the path, the image won’t be saved to a file, but the method will still return a buffer of the screenshot.
How to Screenshot the Full Page in Puppeteer
To screenshot the full page in Puppeteer, set the fullPage
parameter when calling the Page.screenshot()
method.
await page.screenshot({
path: './tmp/screenshot.png',
fullPage: true
})
The fullPage
parameter is set to false by default, but when enabled, Puppeteer will take a full screenshot of the entire scrollable page.
Other helpful screenshot parameters include:
- quality – a numeric valley for image quality between 0 – 100
- omitBackground – screenshot with a transparent background
- encoding – base64 or binary encoding (default is binary)
- clip – give the screenshot specific dimensions or screenshot a specific area of the page
- type – jpeg or png image type (default is png)
- fromSurface – captures from surface instead of view (default to true)
- captureBeyondViewport – capture beyond the viewport (defaults to true)
Now you know how to screenshot an element in Puppeteer and take a full page screenshot. Happy coding!
If you found this guide helpful, check out our Javascript Section for more useful Puppeteer tips, tricks, and guides.