How to Reverse Order of a ForEach Loop in PHP
Learn two methods to reverse the order of a foreach loop in PHP.
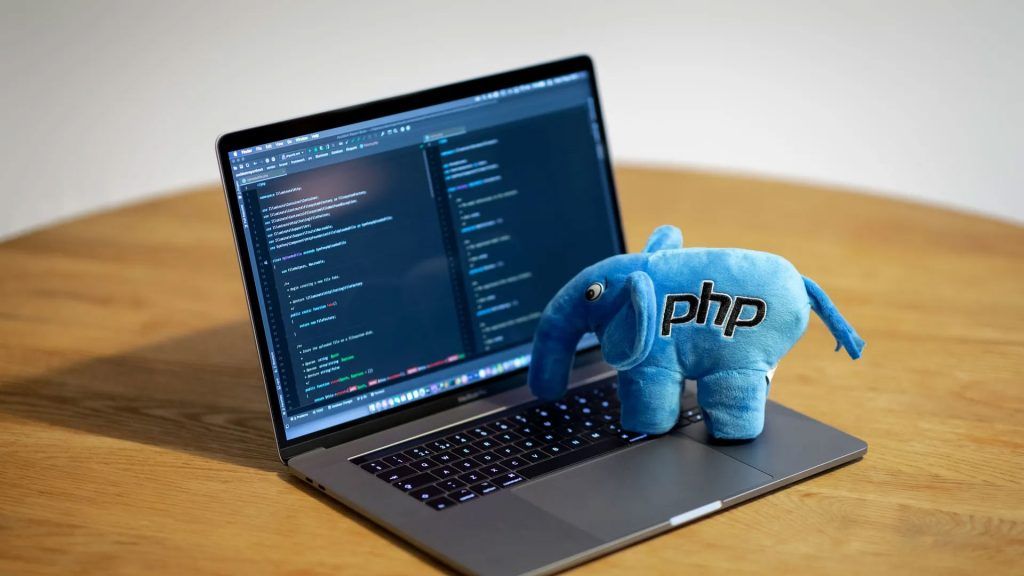
As a programmer, you will quickly become a master of array manipulation. Luckily, the PHP language comes built-in with tons of useful helper functions to help us work with an array. In this tutorial, we will show you two solutions for reversing the order of a foreach loop in PHP. Let’s take a quick look at what we are trying to achieve.
$data = ['a','b','c','d'];
foreach($data as $item) {
echo $item;
}
//OUTPUT: abcd
Here we have a very simple dataset and a foreach loop that traverses it. We want to read this array backwards with our foreach loop so the output would read “dcba.” There are multiple ways to get this done.
How to Reverse Order of a ForEach Loop in PHP
To reverse the order of a foreach loop, you can use the array_reverse method or use a different loop and count through the array backwards. We will look through both solutions in detail here.
Solution 1 – Use array_reverse
PHP comes with a useful function called array_reverse. This method will take in an array and spit out a reversed copy. Let’s take a look at a code example:
$data = ['a','b','c','d'];
foreach(array_reverse($data) as $item) {
echo $item;
}
//OUTPUT: dcba
We can easily use the array_reverse function directly in our foreach parameters to reverse our primary array before spitting out the answers. This solution is very clean and gets the job done but might not be the most optimal.
Since we will always know the size of the array, there isn’t anything preventing us from reading the array backwards instead of having to perform an operation that unnecessarily restructures a copy of it. Let’s look at another example that might be a better option for certain use cases.
Solution 2 – Count the Array Backwards
Instead of rebuilding the array in reverse order, we can also just read it backwards. However, for this solution, we will want to swap out our foreach loop with a while loop. Let’s take a look at a code example:
$data = ['a','b','c','d'];
$index = count($data);
while($index) {
echo $data[--$index];
}
//OUTPUT: dcba
With this solution, we will first get an index by counting the elements in the array. Then we will create a while loop with the index as our parameter. Inside the while loop, we can call our initial data array directly and decrement the index simultaneously. The while loop will conveniently terminate itself once it reaches the end of the array.
Although the second solution doesn’t technically use a foreach loop, it does achieve the same goal in a more efficient way. You should consider using this technique if your project calls for a reverse order of a ForEach Loop in PHP.
We hope you found this guide helpful. Check out our Coding section for more useful tutorials.