How to String Replace the Last Occurrence of a String in PHP
Here is how to perform a more advanced string replace if you need to replace the last occurrence of a substring in PHP.
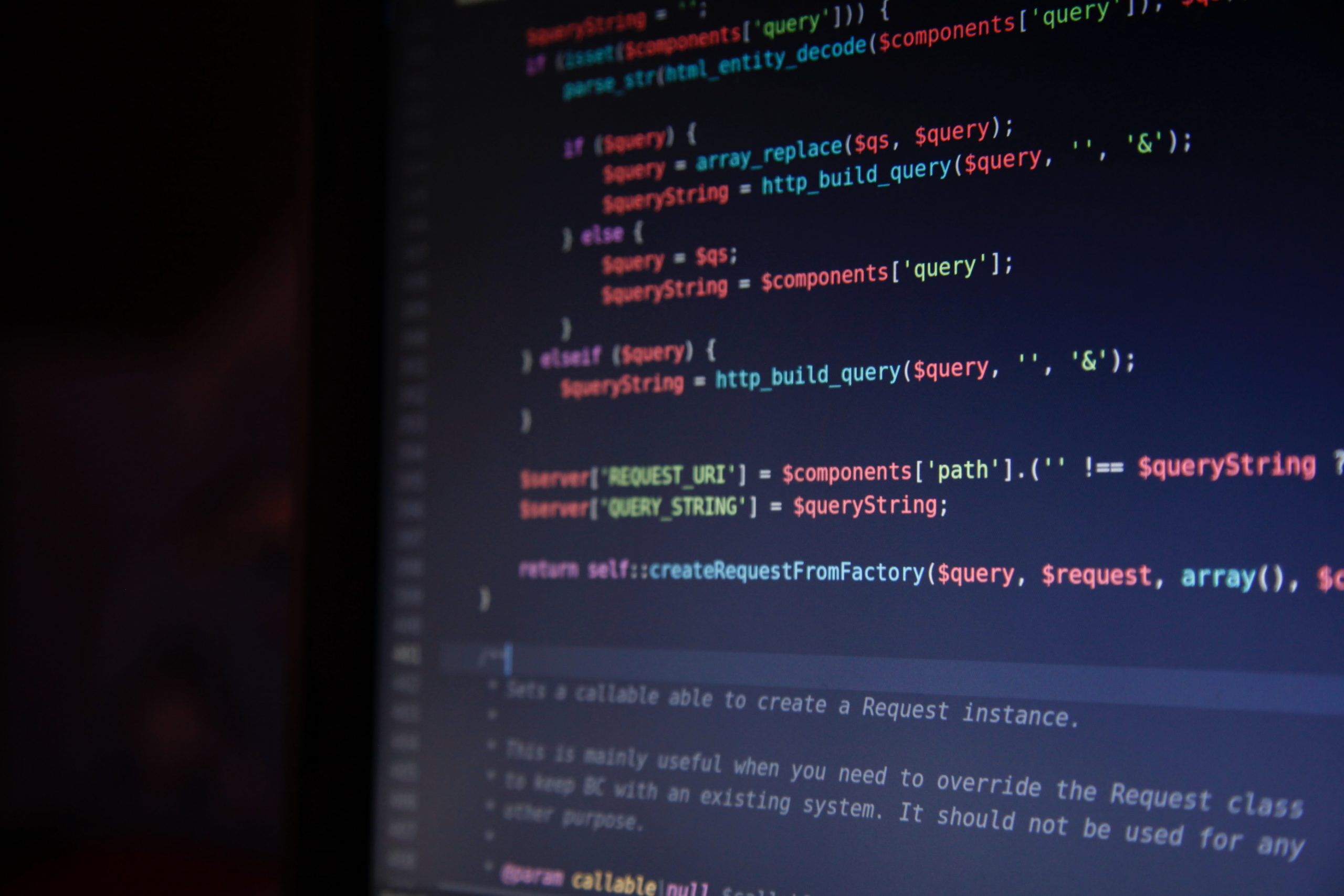
Replacing substrings in PHP is a simple task with the str_replace() function, but what if you wanted to ensure that only the last occurrence of your search term is replaced? Performing this task will require a little more work, but with just a few PHP functions, it can be done without too much code. Let’s learn how to string replace the last occurrence of a string in PHP
How to String Replace the Last Occurrence of a String in PHP (Solution)
To replace the last occurrence of a substring within a string in PHP we will use the strrpos()
and substr_replace()
functions. Let’s look at a code example and then dive into what’s happening.
$searchFor = 'hello';
$replaceWith = 'lets learn!';
$mainString = 'hello from techozu hello';
$stringPosition = strrpos($mainString, $searchFor);
$newString = substr_replace($mainString, $replaceWith, $stringPosition, strlen($searchFor));
echo $newString;
// OUTPUT
// hello from techozu lets learn!
First, we grab the last position index of the substring we want using the strrpos()
function. This function will return the index of our substring or false if it’s not found.
It’s important to note that strrpos()
can return both false and 0. The function will return 0 if the last occurrence of your substring is at the start of the string. Use the ===
operator to test the return value properly.
The strrpos()
function is nearly identical to strpos()
which would find the first occurrence of a substring.
With the last index in hand, we can now use the substr_replace()
function to replace our substring. This method takes in four different parameters:
- The primary string
- The new substring
- The position where you will start replacing
- The number of characters you will replace
To get the number of characters we will be replacing, we use the strlen()
function.
That’s all there is to replacing the last occurrence of a substring in PHP. The solution provided is simple and, most importantly, easy to read and understand at a glance. If you are going to be performing this action repeatedly, it’s recommended to wrap it in a function. Alternatively, you could always use preg_replace()
anytime you’re working with strings and use a regular expression to perform the find and `replace function.
We hope you found this guide helpful. For more useful guides, please check out our PHP coding section.