Remove Elements from an Array by Value in PHP
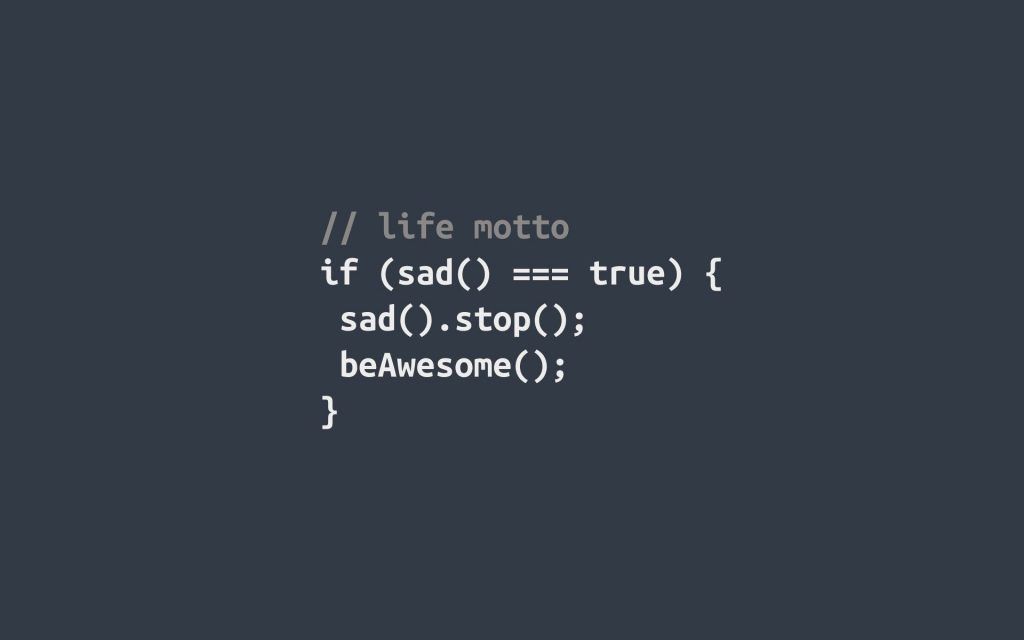
There is no doubt that you will need to learn how to manipulate arrays efficiently when you start coding. Typically first, you will need to remove arrays by their keys. However, you may also need to remove elements by their values. Let’s take a look at how to remove elements from an array by value in PHP.
Solution #1
PHP gives us an excellent function that lets us search our array and find a key with a specific value. That method is array_search. One important thing to note here is that this method will only find and remove the first occurrence of the value in your array. If you need to remove multiple occurrences of the value, then look onto Solution #2
$array = array("foo" => "bar", "hello" => "world");
if (($key = array_search('bar', $array)) !== false) {
unset($array[$key]);
}
In this code, if the array_search method finds a value, it will return its key; otherwise, it will return false. We will then use the returned key, which was populated inside the if statement and unset it from the array.
Solution #2
If we want to remove multiple occurrences from the array, we will need to change our strategy a bit. For this solution, we will use another method called array_keys.
$array = array("foo" => "bar", "hello" => "world", "cash" => "bar");
foreach (array_keys($array, 'bar', true) as $key) {
unset($array[$key]);
}
In this code, the array_keys function returns an array of keys for us. The second parameter in the method is the value we are searching for, and the third enables strict search so that numbers and strings of the same value are not considered the same. We then loop through this array of keys and remove each one with unset.
There you have it. Now you know how to remove elements from an array by value in PHP. Happy Coding!