How to Reference Static Assets in Vue 💡
Learn how to properly reference static and dynamic images in Vue. Make working with images easy!
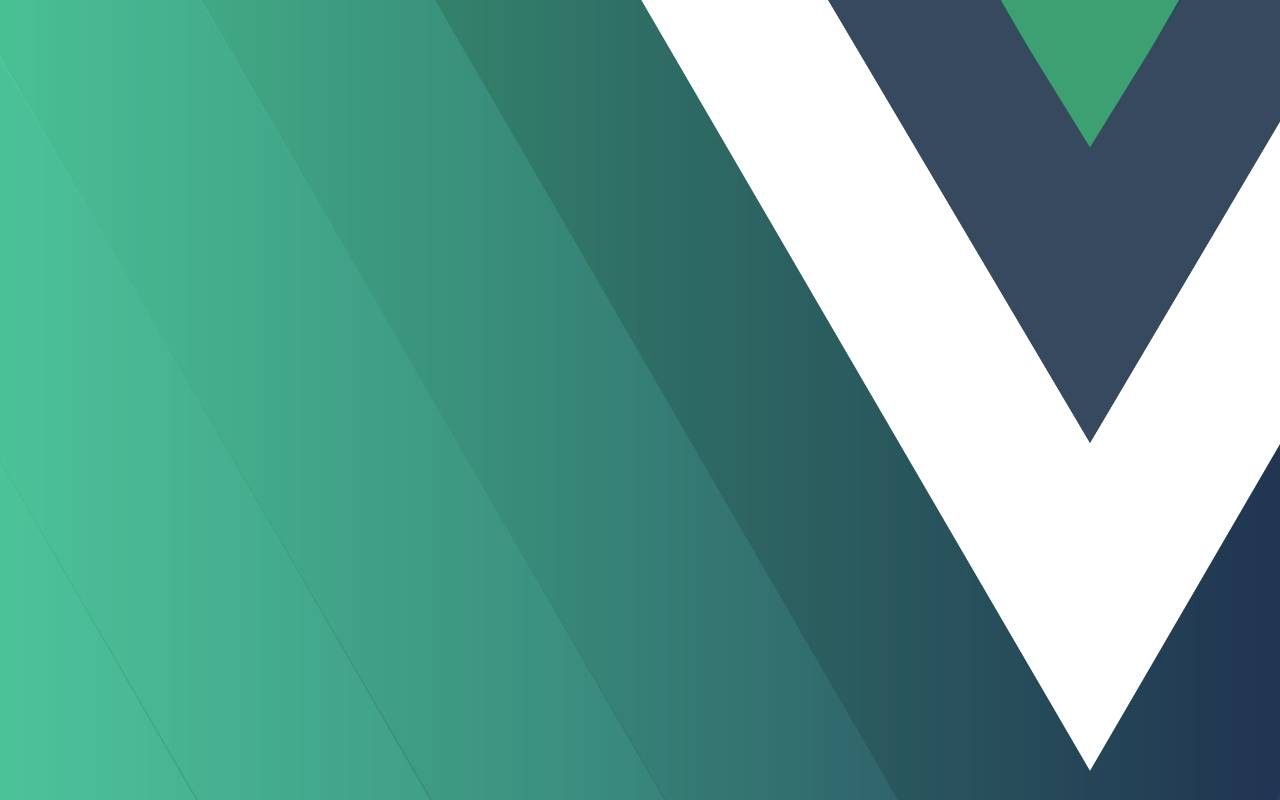
Dealing with images and other assets in Vue can get confusing. Luckily after you understand the flow and learn some helpful reference syntax dealing with images becomes trivial. Here is how to reference static and dynamic assets in Vue.
How to Reference Static Assets in Vue
To reference an image or other files from the assets folder directly from within the template portion of your Vue file, just call the assets folder like so:
<img alt="Techozu" src="@/assets/techozu.png" />
We precede the assets folder where are files are located with @/
. This will reference the src path of the project, allowing us to easily reference the assets folder even if it’s in nested components.
Prepending @/
to assets or components will reference the src folder. Prepending ./
to assets or components will reference the folder your current file resides in.
How to Reference Dynamic Assets Programmatically in Vue
Sometimes we need to reference an asset dynamically within our component code. To do this, we must first require the asset and then pass it to your template. Here are two different examples:
<template>
<img alt="Techozu" :src="logo" />
</template>
<script>
export default {
name: 'App',
data() {
return {
logo: require('@/assets/logo.png'),
}
},
mounted() {},
}
</script>
Here we simply require an asset directly into our data function and then call it using the attribute binding in the template. Let’s look at a more dynamic example
<template>
<img alt="Techozu" :src="getImage('logo.png')" />
</template>
<script>
export default {
name: 'App',
methods: {
getImage(filename) {
let img = require(`@/assets/${filename}`)
return img
},
},
}
</script>
In this particular example, our image is chosen dynamically based on some data fed into our component or generated from within the component itself. A method will require the image based on the filename it’s given. To call the assets, create an attribute binding within your template and reference your method directly.
This technique is helpful if you create an image, icon, button, or other components that may change an image based on different parameters.
You can also require dynamic assets in line with your template by using attribute bindings if you like.
<template>
<img alt="Techozu" :src="require('@/assets/logo.png')" />
</template>
Be cautious about putting too much logic directly into your template. It’s best practice to separate coding logic and markup within your Vue app.
You’re now geared with some helpful techniques for referencing static assets in Vue—both directly and programmatically. Remember always to keep your code clean and readable. Be kind to your future self. 😁
For more helpful Vue guides, tips, tricks, and more head to our Vue Coding Section.