How to Loop through a Javascript Object?
Learn three different methods to iterate over a simple Javascript object.
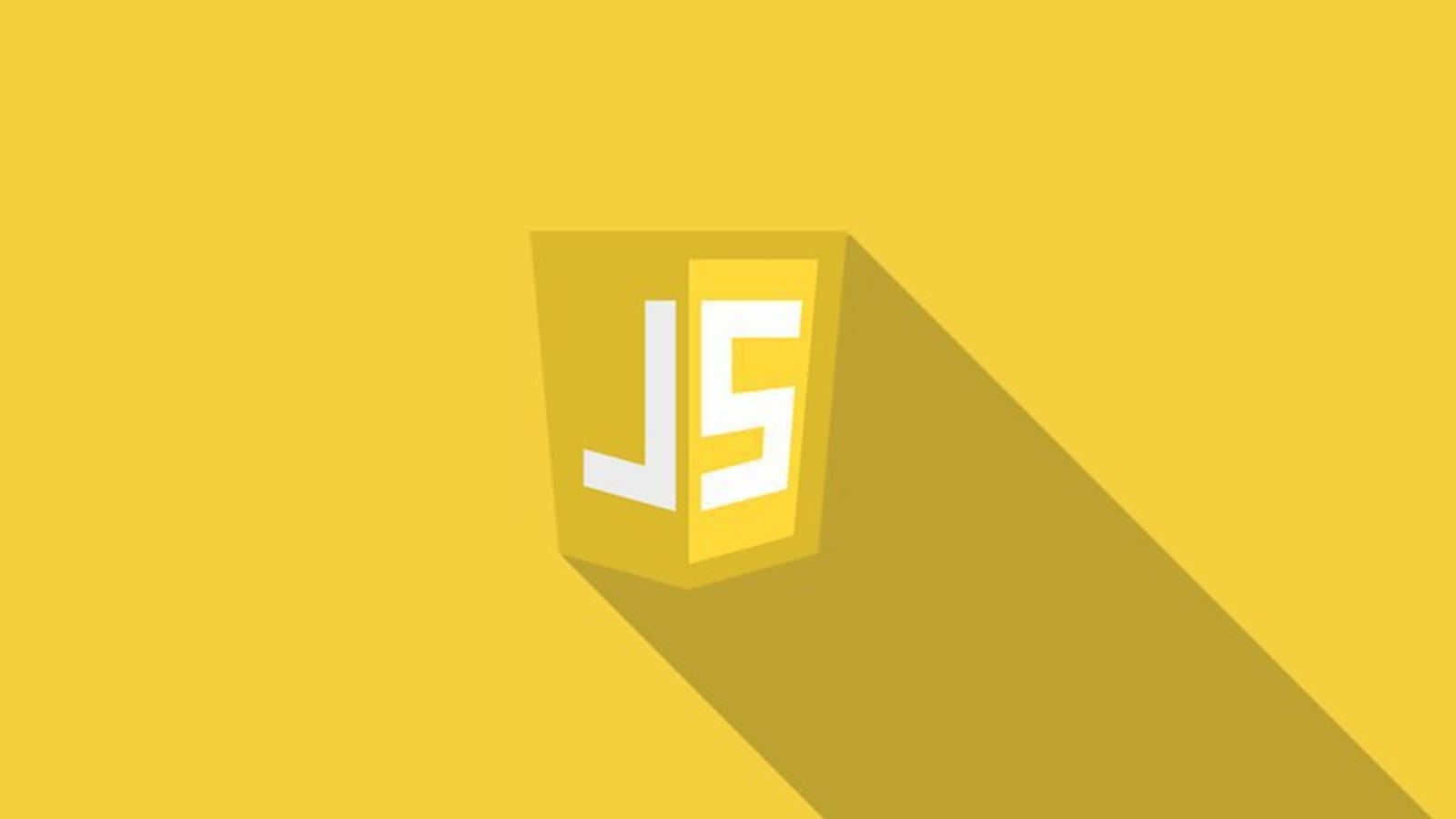
Jumping between Arrays and Objects in Javascript can quickly get very confusing. Not all operations you use to manipulate objects will work on arrays and vice versa. A basic task when working with Javascript objects is the ability to loop through their keys or values. The intuitive method for this spits out errors but don’t fret. We have multiple solutions for you on how to loop through a Javascript object.
How to Loop through a Javascript Object?
There are multiple methods to loop through a Javascript object. We will focus on using for-in, Object.keys(), and Object.entries()
Using Object.entries()
The Object.entries() method will return an array of enumerable key-value pairs. We can perform a for-of loop on this array to get each key entry and its value in simple and compact syntax. Here is an example:
let myObj = {
a: 'hello',
b: 'from',
c: 'techozu'
}
for ([key, value] of Object.entries(myObj)) {
console.log(key, value)
}
//OUTPUT
// a hello
// b from
// c techozu
The returned array from Object.entries() alone would look something like this:
let myObj = {
a: 'hello',
b: 'from',
c: 'techozu'
}
console.log(Object.entries(myObj))
//OUTPUT
// [ [ 'a', 'hello' ], [ 'b', 'from' ], [ 'c', 'techozu' ] ]
Please be aware that Object.entries() is not supported on any version of Internet Explorer. So unless you use a compiler like Babel to package your Javascript for the web, you may want to use a different solution.
Using a for-in loop
If you try and perform a standard for-of operation on an object, you will receive an error saying the variable “is not iterable.” Instead of the for-of loop, we want to use the for-in loop. The for-in loop will loop through each key in the object. We can then use the key provided in the loop to pull the values. Here is an example:
let myObj = {
a: 'hello',
b: 'from',
c: 'techozu'
}
for (key in myObj) {
if (myObj.hasOwnProperty(key)) {
console.log(key, myObj[key])
}
}
//OUPTUT
// a hello
// b from
// c techozu
Verifying your key before performing any operations with the hasOwnProperty() method is a good idea. This will ensure you are not performing actions on any prototype properties that may be associated with the object.
Using Object.keys()
As an alternative to the for-in loop we can use a different object method called Object.keys(). This method will return an array of keys which we can then loop through using the for-of loop. Here is an example:
let myObj = {
a: 'hello',
b: 'from',
c: 'techozu'
}
for (key of Object.keys(myObj)) {
console.log(key, myObj[key])
}
//OUPTUT
// a hello
// b from
// c techozu
Using Object.keys() will also help ensure that we are iterating on the object keys only and not any prototype keys.
Now you know how to loop through a Javascript object, and you have a few options to choose from based on your use case and syntax preferences. It’s important to note that the solutions listed above will only loop through the root keys of the object. If you have a nested object and need to perform a deep traversal, you may want to use one of the methods above recursively.
We hope you found this guide helpful. For more useful Javascript and coding guides, take a look through our Javascript section.