How to Find and Update Object in Javascript Array
Power up your Javascript by learning how to find and update an object in an array.
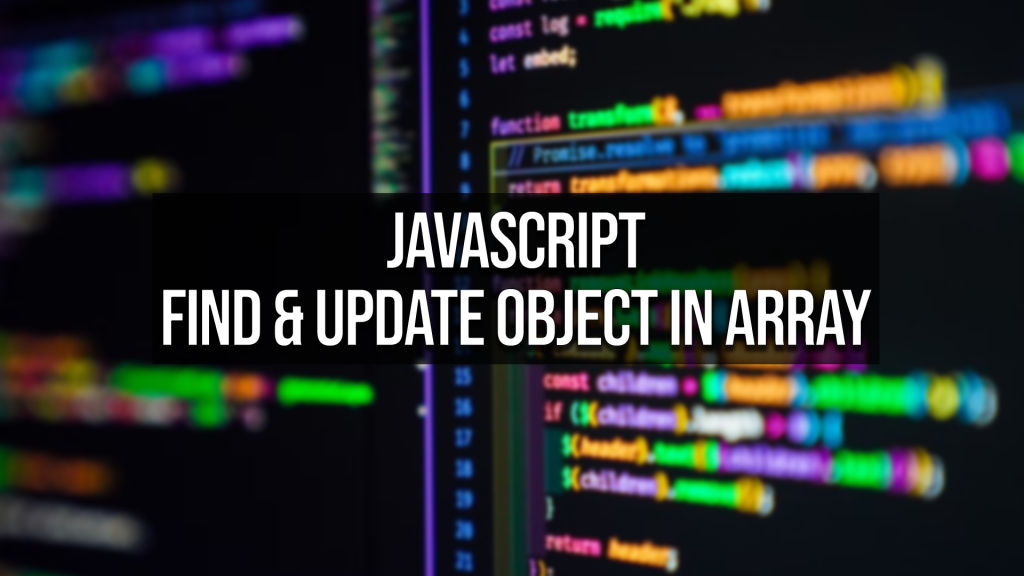
If you are going to be a Javascript developer, you will need to get comfortable with arrays and objects. More specifically, you will need to learn how to manipulate objects within arrays. Luckily we are here to help. Read on to identify our problem and multiple solutions for tackling it.
The Problem – Find and Update Object in Array
Suppose we have an array of objects:
var inventory = [{item: 'shirt', quantity: 10}, {item: 'pants', quantity: 4}]
We want to find an item in this array and update its quantity. And we would like to do this without copying the entire array over to a new variable. Since we are just dealing with an array at the basic level, we can use the findIndex method to search for the index of the object we need.
The Solution – findIndex
Using findIndex is simple. Here is the code with the breakdown below:
var itemIndex = inventory.findIndex(x => x.item == 'shirt')
var item = inventory[itemIndex]
item.quantity = 2
inventory[itemIndex] = item
In the first line, we use findIndex on the array and search for the item we want. In this case, it’s a ‘shirt,’ but it would be some sort of unique identifier in a realistic scenario. The return is the index position of the object within our array. We can now pull that object into a variable and update any field we like, seen in lines two and three. Finally, we write our new object back into the original array in line four.
We can also shorten this solution by using the spread operator when we write back to the array.
var itemIndex = inventory.findIndex(x => x.item == 'shirt')
inventory[itemIndex] = {...inventory[itemIndex], quantity: 2}
Above, you can see that we skipped writing our object to a variable first and just wrote the updated object directly to the array.
This solution is short and clean, but it assumes that you are searching for an object with a unique identifier inside your array. If you have a repeat object within the array, you will need to perform your operation within a loop. Here is an example:
inventory.forEach(x, index) => {
if(x.item == 'shirt') {
inventory[index] = {...x, quantity: 2}
}
}
Of course, you can use any looping syntax you like for this operation.
We hope you found this guide helpful. If you have any questions about the code above or javascript questions in general, please feel free to reach out.