How to Split Messages in Discord.js
Avoid the 2000 character limit by learning how to split messages in Discord.js
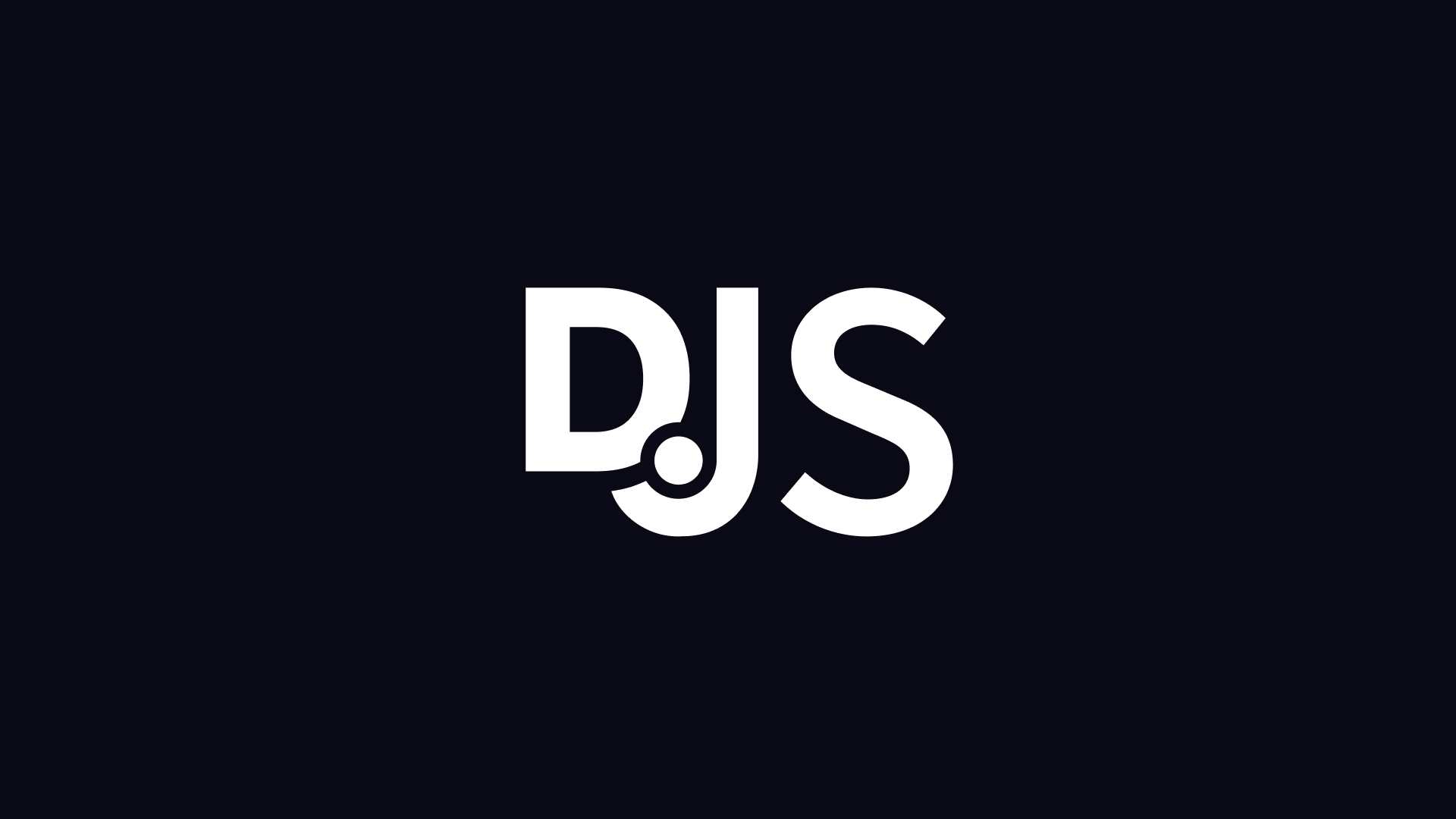
Sending messages to a Discord channel or user using Discord.js is a simple task. However, if you try to send a long message, you might run into an error stating that your message is over 2000 characters. Discord.js used to have built-in functions to avoid this error, but they have since been depreciated. Here is how to split messages in Discord.js.
How to Split Messages in Discord.js
Use the following code to split your message into chunks and then send multiple individual messages:
const messageChunks = splitMessage(msg, 2000)
for (chunk of messageChunks) {
await YourDiscordChannel.send(chunk)
}
function splitMessage(str, size) {
const numChunks = Math.ceil(str.length / size)
const chunks = new Array(numChunks)
for (let i = 0, c = 0; i < numChunks; ++i, c += size) {
chunks[i] = str.substr(c, size)
}
return chunks
}
In this code, we write a function that takes in a string and a split size. You can hardcode the split size to 2000 if you wish, but in the example, it’s left as a variable to make the function more dynamic.
When a section of code performs a particular task that could be reusable, put it into its own function–even if you don’t foresee yourself using it multiple times.
We first get the number of message chunks we need by getting the string length, divided by the split size, and using the Math.ceil
function to round the number up. We then create an array to hold the chunks and loop through the array. Loop with two variables, one for the chunk index and the other for the substring index, which we will use with the substr() function to get our chunked string.
Then, we return the array of chunks and loop through it again elsewhere in our code to send the actual messages.
Split Parameter is Deprecated
Discord.js originally allowed you to add a {split: true}
parameter to your message to handle a long message split automatically. This code was deprecated in version 13.
//Deprecated Code
YourDiscordChannel.send(message, {split: true});
You can install version 12 of Discord.js if you need this functionality.
splitMessage Utility is Deprecated
When the split parameter was deprecated, it was replaced by the Util.splitMessage
helper in version 13. However, this helper was also deprecated and removed in version 14.
//Deprecated Code
const { Util } = require('discord.js')
const messageChunks = splitMessage(msg)
for (chunk of messageChunks) {
await YourDiscordChannel.send(chunk)
}
If you want to use the Util.splitMessage helper, you will need to install version 12 of DiscordJS.
How to Use an Old Version of Discord.js
If you would like to work with an older version of Discord.js that has the built-in capability to split messages, you can use npm
.
npm install [email protected]
Change the version number after the @ sign to anything you like, and that version of Discord.js will be installed.
You now have everything you need to split messages in Discord.js, including older versions of Discord to use previously built-in functions. Happy coding!
If you want more JavaScript coding tutorials, head to our JavaScript Section.