How to Sort an Array of Objects By Value in Javascript
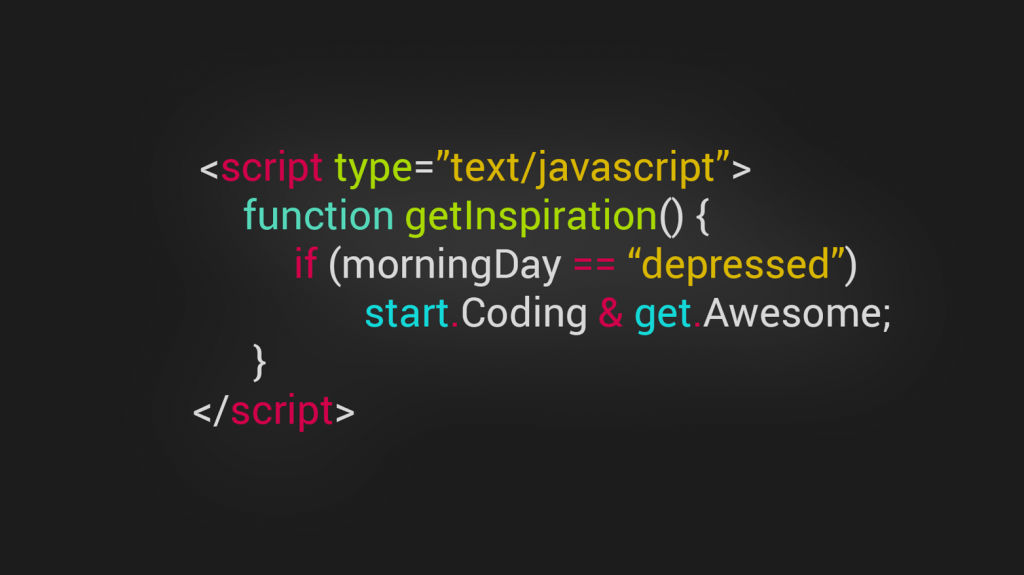
After filling our Javascript arrays up with data, we may need to sort that data constructively. Sorting a regular array in Javascript is pretty straightforward, but what if you have an array of objects. That makes things slightly more complex. To sort that, we will need to compare the values recursively. Luckily javascript makes this easy. Let’s take a look at how to sort an array of objects by value in javascript.
Sorting an Array of Objects by Value
Let’s imagine that we have an array of objects holding a list of employees.
const employees = [
{ firstName: 'Bill', lastName: 'Cooper', age: 43},
{ firstName: 'James', lastName: 'River', age: 23},
{ firstName: 'Jill', lastName: 'Randell', age: 34},
{ firstName: 'Phillip', lastName: 'Morgan', age: 38},
]
We want this list to be ordered by age from youngest to oldest. How can we achieve this?
Well the answer is actually simple. We can use a built-in Array method called sort(). Let’s take a look at how to use it.
employees.sort((a, b) => (a.age > b.age) ? 1 : -1)
Our function inside sort is called the “compareFunction”. It will compare one element with another. In this case, it will see if a is greater than b. That example will order the array in ascending order with the youngest age at index 0.
If we output our array it will now look like this:
[
{ firstName: 'James', lastName: 'River', age: 23},
{ firstName: 'Jill', lastName: 'Randell', age: 34},
{ firstName: 'Phillip', lastName: 'Morgan', age: 38},
{ firstName: 'Bill', lastName: 'Cooper', age: 43},
]
To reverse the order and have it be descending, we would just use this function.
employees.sort((a, b) => (a.age < b.age) ? 1 : -1)
Now the oldest age has the 0 index. You can use the same method if you want to sort it alphabetically. Simply change the property you are going to compare. For more details on the inner workings of the sort() method check out its official documentation. Happy Coding!