How to Replace All Occurrences of a String?
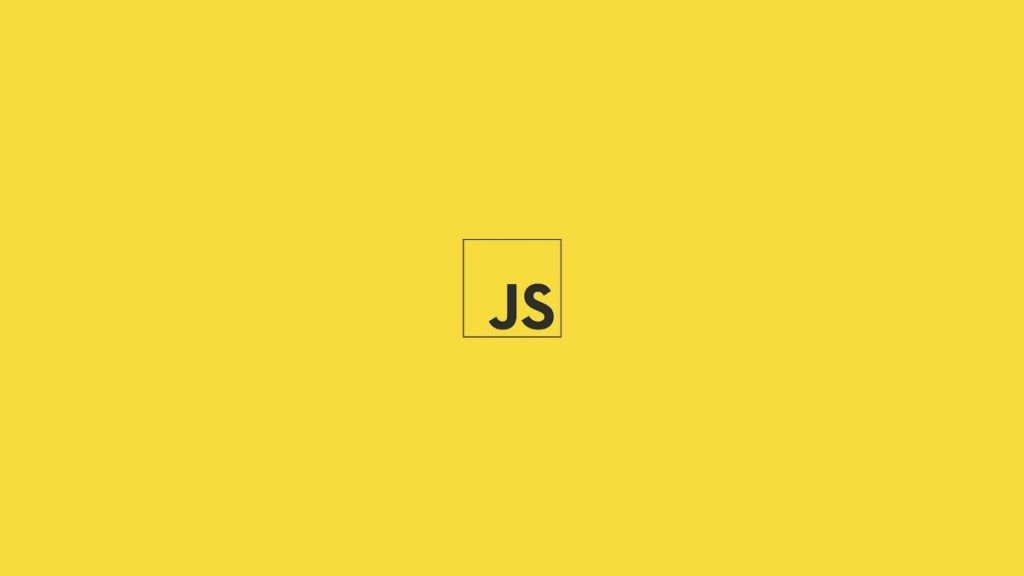
If you are working with JavaScript, you may come across a situation where you need to find and replace all occurrences of a string within another string. Luckily JavaScript gives us some clean solutions to this problem.
The Problem
Let’s says we have a string.
Input String
“This is a String. This is the Best String.”
We want to take this string and remove all instances of “is” from our string. Here is our intended output.
Output String
“This a String. This the Best String.”
Solution #1
In new browsers, we can use the new replaceAll string prototype. This method gives us a clean, one-liner solution. The first parameter is the substring you are looking for, and the second parameter is what you are replacing it with.
var str = "This is a String. This is the Best String.";
var answer = str.replaceAll(" is","")
Be careful. This code does not take individual words into account. So if your substring is part of a word, then it will be replaced. In that case, make sure to include spaces like in the example above.
Solution #2
Another solution that will work on most browsers is using the replace string prototype together with a regex expression. The first parameter is the regex expression to find all the occurrences of your substring. We make sure to use the g modifier to get all the matches. The second parameter is what we will be replacing the string with.
var str = "This is a String. This is the Best String.";
var answer = str.replace(/ is/g,"")
There you have it, two nice and easy ways to find and replace all string occurrences within another string.
Check out our other guide on how to check if a string contains another string.