How to Check if an Array has More than One Element in PHP
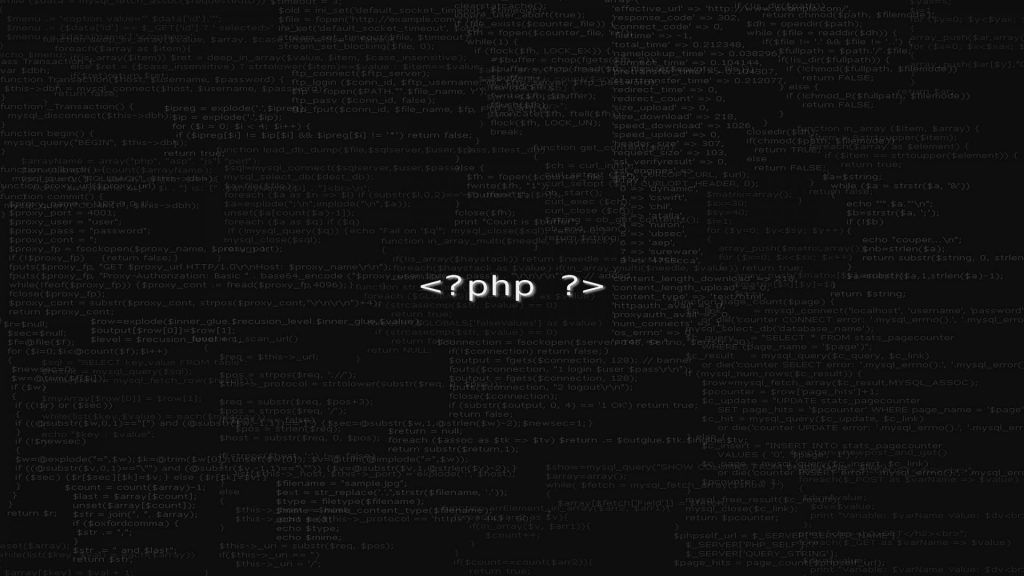
An array is a data structure of elements. In PHP, an array is primarily a map of ordered elements. It may be useful to know how to tell how many elements are in a particular array or to be able to find out if an array has more than one element. We will find out how to check if an array has more than one element in this how-to.
Solution #1
There are multiple solutions to this problem. One way is to use the PHP count method. The count methods will take an array in as an argument and give you back how many total elements are in it. All we have to do is see if this count is greater than one, and we have our answer.
if (count($your_array) > 1) {
//Do Something
}
Solution #2
Our second solution is to use the sizeof method in PHP. This method is actually just an alias for the count method. So it performs the same task in the same way.
if (sizeof($your_array) > 1) {
//Do Something
}
Solution #1 is the preferred way to check if an array has more than one element in PHP. The count method will appear cleaner in your code as in some languages, sizeof refers to the amount of allocated memory, which can make coming back to your code in the future a bit more confusing.
To make your code a bit more robust is might also be a good idea first to check that your array is, in fact, an array and not some other time. To do this, we can use the is_array method.
if (is_array($your_array) && count($your_array) > 1) {
//Do Something
}
However, this verification may only be necessary for some instances.
So that’s it, now you know how to check if an array has more than one element in PHP. You can easily modify this code to check if an array has more than any amount of elements. Happy Coding!