How to Format Date Strings in PHP
Learn how to format date strings in PHP by mastering two simple functions.
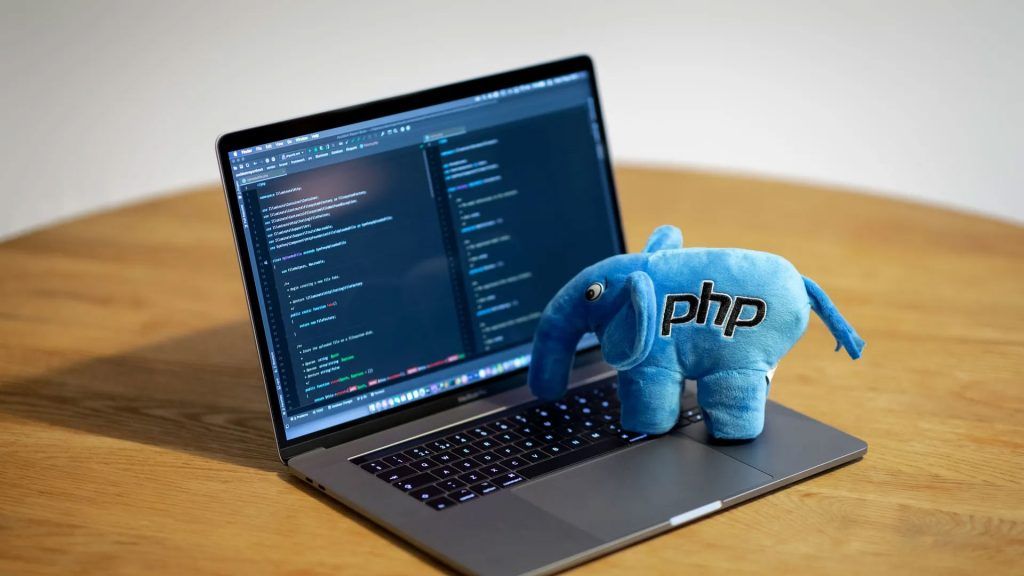
Learning to work with date and time strings is a must in any programming language. Luckily PHP makes formatting date strings and also generating the needed Unix timestamps very easy. Here is how to format date strings in PHP.
How to Format Date Strings in PHP
To format date strings, you can use a combination of the built-in date()
and strtotime()
methods. Let’s take a look at an example:
$timestamp = strtotime("2022-10-05T04:00:00.000Z"); //Returns UNIX Timestamp
$formattedDate = date('F j, Y', $timestamp);
echo $formattedDate;
// OUTPUT
// October 5, 2000
strtotime()
is available in PHP 4+ and date()
is available in PHP 5.2+
The strtotime()
method is a powerful PHP function that will parse a textual DateTime description directly into a Unix timestamp. Here are some examples of values you can pass into the strtotime()
method.
- strtotime(“-2 days”)
- strtotime(“+5 hours”)
- strtottime(“20 October 2022”)
- strtotime(“next Friday”);
- strtotime(“2022-10-05T04:00:00.000Z”);
- strtotime(“now”)
You can read more about valid DateTime formats in the official documentation.
With the timestamp in hand, you can use the date()
method to format your timestamp. The date()
function takes in a format string and your Unix timestamp. You can read through all of the possible format characters in the official documentation, but here are some of the most popular ones.
- date(‘F j, Y’, $timestamp) // October 6, 2022
- date(‘n/j/Y’, $timestamp) // 10/6/2022
- date(‘F j Y’, $timestamp) // October 6 2022
- date(‘Y-m-d, $timestamp) // 2022-10-06
If your input datetime string is already a Unix timestamp, you can bypass the use of the strtotime()
function. You can also omit the Unix timestamp parameter from the date()
function to use the current timestamp.
date('F j Y'); //Current Formatted Time
Using the date()
method without a timestamp parameter is the equivalent of using Date.now() in JavaScript.
You now have everything you need to format Date Strings in PHP. One of the most common use cases will be to format date ISO string when importing data from a database or various microservices.
Check out more useful PHP tips, tricks, and guides in our PHP Coding Section.