Fix replaceAll is not a function in Node JS
Easily fix the “replaceAll” is not a function error in NodeJS with the following solutions.
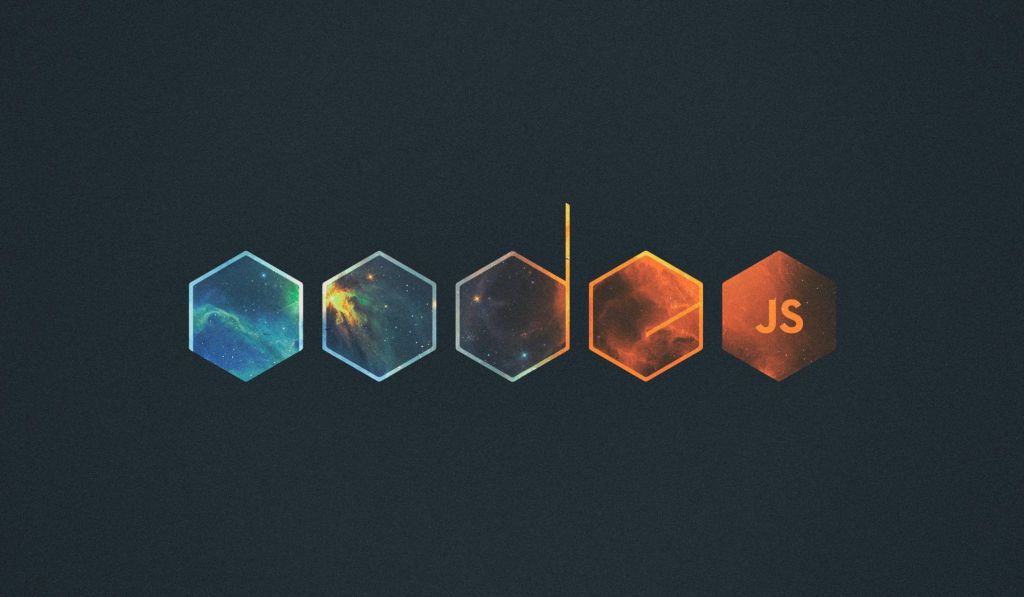
The replace function is one of the most used string prototypes in Javascript. It allows you to replace the first occurrence of a specific substring with anything you like. If you need to replace all instances of that substring, you may be inclined to use another function called replaceAll. That is, of course, if you’re in the NodeJS environment where the function may not work. Let’s take a look at an example and see what’s going on.
let input = "00aa00bb00cc00dd"
let output = input.replaceAll('00', '');
console.log(output)
//OUTPUT
//TypeError: input.replaceAll is not a function
The code above works flawlessly on all major browsers (except Internet Explorer). However, when you try to use it inside a NodeJS version 14 or lower environment, it yells at you, saying that replaceAll is not a function. So what gives? In NodeJS, “replaceAll” is not a native string prototype (below Node version 15). Hence you get the above error. Let’s take a look at some alternatives below.
How to replaceAll in Node JS
The replaceAll method is available in Node versions 15 and up. If you’re getting an error, you are likely using an older Node version. One option is upgrading your Node environment. However, another alternative to “replaceAll” in Node JS is the standard “replace” function with a regular expression. Let’s take a look at how to use it and also how we can add our own replaceAll function for repetitive use.
Solution 1 – Use replace with regex
The replace string prototype will allow us to replace the first occurrence of a string within another string. However, we can also feed the replace function a regular expression. Let’s look at an example.
let input = "00aa00bb00cc00dd"
let output = input.replace(/00/g,'')
console.log(output)
//OUTPUT
//aabbccdd
Our target string is put between two forward slashes followed by the g flag to complete the regex. If you remove the g flag, the regex will once again just replace the first occurrence.
Solution 2 – Create your own replaceAll prototype
In the following example, we will add a new method to the String prototype. This will allow us to use the replaceAll function on any string within our code.
String.prototype.replaceAll = function (target, payload) {
let regex = new RegExp(target, 'g')
return this.valueOf().replace(regex, payload)
};
let input = "00aa00bb00cc00dd"
let output = input.replaceAll('00', '');
console.log(output)
//OUTPUT
//aabbccdd
Inside our replaceAll prototype, we use the standard replace function with a regular expression from Solution 1.
Solution 3 – Update to Node 15+
Depending on how your development and production environments are set up, the simplest solution may be to update your Node version to 15 or higher. This, of course, could potentially cause other issues in other parts of your code. It’s always a good idea to install Node using a version manager such as NVM (Node Version Manager). That way, you can easily install a current version of Node but revert back if necessary. It will also allow you to match your final production environment more closely.
We hope you found this guide helpful. For more useful Node tutorials, please check out our Javascript section.