How to exit a forEach Loop in Javascript
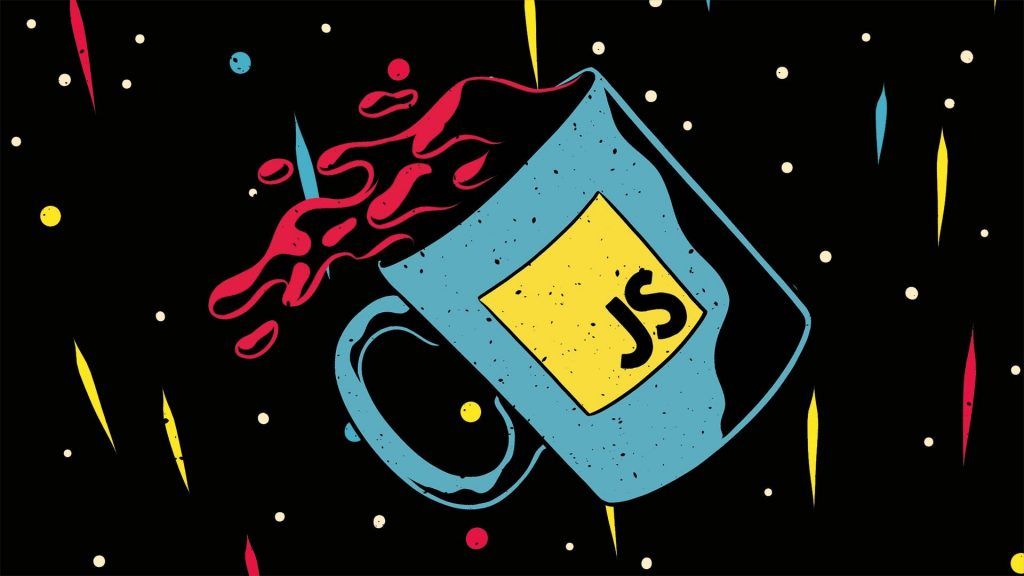
It goes without saying that the majority of coding is dealing with loops. There are all kinds of loops with all sorts of different syntaxes. It’s challenging to make sense of it all, but part of becoming proficient in programming is knowing what syntax to use, especially when there are multiple options. One loop in javascript is the forEach loop. It’s used to iterate over arrays. It provides a nice syntax, but issues arise when we try to get a bit more complicated, such as breaking out of the loop. Let’s learn how to exit a forEach loop in javascript.
Table of contents
How to Exit a forEach Loop in Javascript
Officially, there is no proper way to break out of a forEach loop in javascript. Using the familiar break syntax will throw an error. If breaking the loop is something you really need, it would be best to consider using a traditional loop.
Let’s look at the following code snippet.
let data = [
{name: 'Rick'},{name: 'Steph'},{name: 'Bob'}
]
data.forEach(obj => {
console.log(obj.name)
if (obj.name === 'Steph') {
break;
}
})
You would expect the code to stop executing after it finds the name “Steph”; however, it throws an Unsyntactic break error.
Solution
As in most things in programming, there is, of course, a workaround. We can throw and catch an exception while iterating our array. Let’s take a look at how we could achieve this.
let data = [
{name: 'Rick'},{name: 'Steph'},{name: 'Bob'}
]
try {
data.forEach(obj => {
console.log(obj.name)
if (obj.name === 'Steph') {
throw 'Break';
}
})
} catch (e) {
if (e !== 'Break') throw e
}
As you can see here, we wrapped our forEach statement in a try catch block. Where we would typically do a break, we perform a “throw ‘Break’“
We then jump down to our catch and check what our error is. If it’s anything other than “Break“, we throw it up the error chain. Otherwise, we continue with our code execution.
Although this solution works, it’s not very clean. Let’s take a look at a few better alternatives.
Alternative #1: for…of loop (Preferred)
The for…of loop would be the preferred solution to this problem. It provides clean easy to read syntax and also lets us use break once again.
let data = [
{name: 'Rick'},{name: 'Steph'},{name: 'Bob'}
]
for (let obj of data) {
console.log(obj.name)
if (obj.name === 'Steph') break;
}
Another added benefit of using the for…of loop is the ability to perform await operations within it. We would not be able to use the await syntax within a forEach loop.
Alternative #2: every
We can also use the “every” array prototype. Let’s take a look at that code snippet.
let data = [
{name: 'Rick'},{name: 'Steph'},{name: 'Bob'}
]
data.every(obj => {
console.log(obj.name)
return !(obj.name === 'Steph')
})
The every prototype will test each element against our function and expect a boolean return. When a value is returned false, the loop will be broken. In this case, we inverse our name test and return false when the name is equal to “Steph”, the loop breaks, and we continue our code.
Alternative #3: some
Very similar to the every prototype, we could also use the “some” array prototype. The some prototype is almost identical to the every prototype. The only difference is that a true return will break the loop. Let’s take a look at the code.
let data = [
{name: 'Rick'},{name: 'Steph'},{name: 'Bob'}
]
data.some(obj => {
console.log(obj.name)
return (obj.name === 'Steph')
})
You can see that the function returns true when it reaches the “Steph” name and breaks the loop. Whether you choose some or every is entirely dependent on what you believe to be the most readable.
You now know how to exit a forEach loop in Javascript. You have multiple alternative solutions and also the primary workaround. Choose the option that fits your use case the best. Remember, code readability is very important, so choose wisely. Happy Coding!