How to Get Elements between Indexes in Javascript Array
Learn how to get a range of items from a Javascript array.
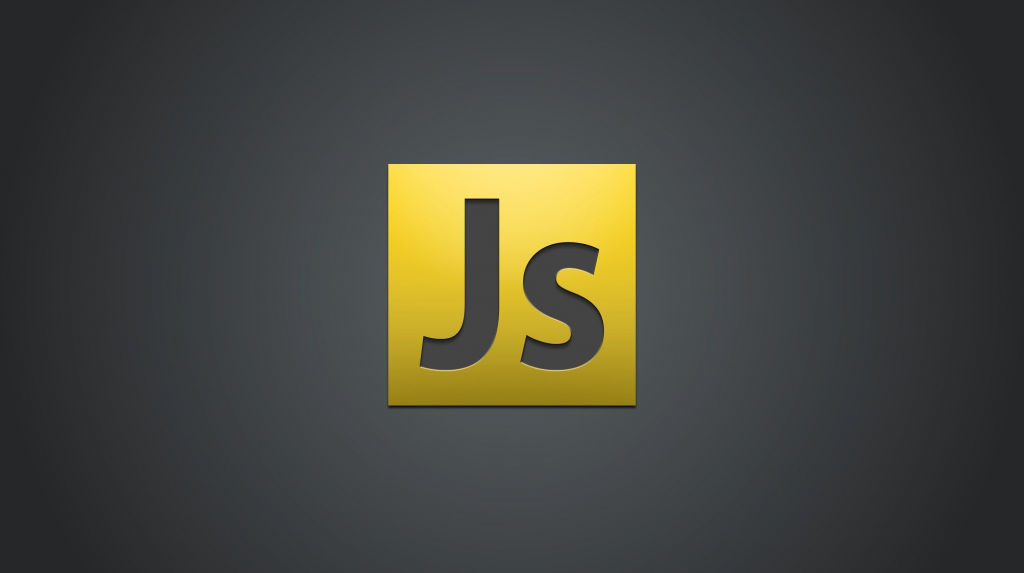
If you want to become a proficient programmer, you will need to master the use of the array. Nearly every Javascript project will require the use and manipulation of arrays with various element types. One operation you may need to perform is retrieving a range of elements from an array. Here is how to get elements between two indexes in a Javascript array.
How to Get Elements between Indexes in Javascript Array
Retrieving elements between two indexes in an array is a simple operation. This process can also be referred to as getting a “range” of items. We will be using the slice prototype as our solution here.
Solution: Slice Prototype
var YourArray = ['a','b','c','d','e','f','g','h','i','j'];
var ArrayRange = YourArray.slice(0, 3);
//Output: ["a", "b", "c"]
Our solution uses a built-in array prototype called a slice. Slice can take two indexes and will return an array of elements between those index values. In our example, we give it a starting value of index 0, which is where we will start including elements. Our end value of index 3 is where we will begin excluding values. Notice that it counts up to and not including the end index, so your return indexes will be 0,1,2 in our example outputting A, B, and C.
If we wanted to retrieve D, E, and F, our code would look like this:
var YourArray = ['a','b','c','d','e','f','g','h','i','j'];
var ArrayRange = YourArray.slice(3, 6);
//Output: ["d", "e", "f"]
The slice method can also take a single start value or negative values. Let’s take a look at a few examples.
var YourArray = ['a','b','c','d','e','f','g','h','i','j'];
var ArrayRange = YourArray.slice(6);
//Output: ["g", "h", "i", "j"]
A single positive value will set a starting index and output everything from there to the end of the array.
var YourArray = ['a','b','c','d','e','f','g','h','i','j'];
var ArrayRange = YourArray.slice(-2);
//Output: ["i", "j"]
A single negative value will offset the array and output values from the end.
var YourArray = ['a','b','c','d','e','f','g','h','i','j'];
var ArrayRange = YourArray.slice(0, -2);
//Output: ["a", "b", "c", "d", "e", "f", "g", "h"]
If we have used a negative value for the end index, we will offset the end and include everything except the last two values.
Caveats: The Reference Problem
One point that’s important to understand is that the slice operation does not change the original array. It will make a “shallow copy” of element references into a new array. Everything works as you would expect if you work with Strings, Numbers, or Boolean values in your original array. However, if you are working with an array of objects, both arrays will share the same references. If an element changes on the original array, those changes will be reflected in your sliced array.
Let’s take a look at an example:
var YourArray = [{id: 'a'},{id: 'b'},{id: 'c'}]
var ArrayRange = YourArray.slice(0, 2);
console.log(ArrayRange)
YourArray[1].id = 'what?'
console.log(ArrayRange)
//Output [{id: "a"},{id: "b"}]
//Output [{id: "a"},{id: "what?"}]
Notice that even those we changed in id value in our original array, our new array also changed. This may be a counter-intuitive behavior. If you know that your original array won’t change, this might not be an issue, but this reference issue can lead to some difficult debugging if your original array might change.
Here is how we can avoid this issue:
var YourArray = [{id: 'a'},{id: 'b'},{id: 'c'}]
var ArrayRange = JSON.parse(JSON.stringify(YourArray.slice(0, 2)));
console.log(ArrayRange)
YourArray[1].id = 'what?'
console.log(ArrayRange)
//Output [{id: "a"},{id: "b"}]
//Output [{id: "a"},{id: "b"}]
To avoid the reference issue, we simply make a clone of the array using the JSON stringify and parse methods. This will give us a new array that does not hold any references to the original. There are multiple different ways of achieving this cloning operation.
We hope you found this guide helpful. If you need more assistance, please check out our Javascript coding section. Happy Coding!