How to Detect a Mouse Drag in JavaScript
Use the following code to differentiate between a mouse click and a mouse drag in JavaScript.
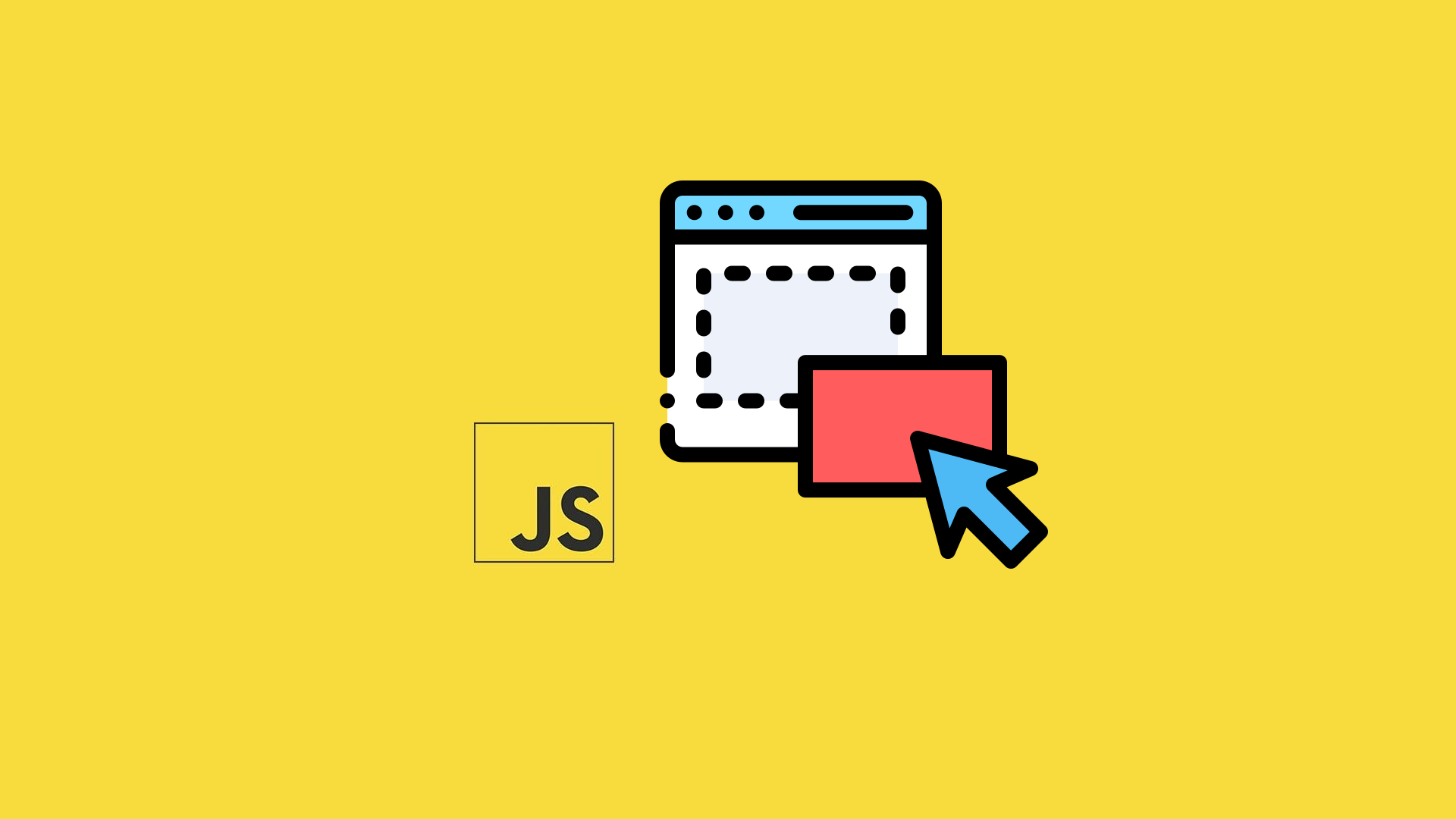
Working with mouse events is one of JavaScript’s most basic use cases. Detecting clicks is trivial, but it gets a little bit more complicated when trying to differentiate between click and drag events. Hopefully, the code samples below will steer you in the right direction for your project.
Identify a Mouse Drag vs. Mouse Click
Detecting a mouse drag will require the use of three different mouse events, mousedown
, mousemove
, and mouseup
. Let’s take a look at a code sample and then break it down.
let drag = false
let downListener = () => {
drag = false
}
let moveListener = () => {
drag = true
}
let upListener = () => {
if (drag) {
console.log('Drag Detected')
} else {
console.log('Click Detected')
}
}
window.addEventListener('mousedown', downListener)
window.addEventListener('mousemove', moveListener)
window.addEventListener('mouseup', upListener)
The idea is very straightforward:
- Create a boolean variable that will store the drag state between clicks
- On mouse down clear the boolean
- On mouse move set the boolean to true
- On mouse up, check the boolean. If true, the mouse was dragged; if false, it was just clicked.
The code above sets the event listeners on the entire viewable window, but you can also just set the listeners to a specific element.
let element = document.getElementById('some_element')
element.addEventListener('mousedown', downListener)
element.addEventListener('mousemove', moveListener)
element.addEventListener('mouseup', upListener)
Check out the JSFiddle if you want to play around with the code.
In many cases, releasing or removing the event listeners may also be necessary. Doing so is very simple.
window.removeEventListener('mousedown', downListener)
window.removeEventListener('mousemove', moveListener)
window.removeEventListener('mouseup', upListener)
Set a Mouse Drag Threshold
The code above will detect a mouse drag fine, but it’s very sensitive. If a user intends to click the mouse but moves their mouse slightly, it will still detect a drag. For this reason, it’s a good idea to introduce a threshold when detecting a drag. The threshold is simply a minimum distance the mouse needs to move to be considered a drag. Let’s look at a coding example.
let drag = false
let threshold = 5
let startingX
let startingY
let downListener = (e) => {
drag = false
startingX = event.pageX
startingY = event.pageY
}
let moveListener = (e) => {
const moveX = Math.abs(e.pageX - startingX)
const moveY = Math.abs(e.pageY - startingY)
if (moveX >= threshold || moveY >= threshold) {
drag = true
}
}
let upListener = () => {
if (drag) {
console.log('Drag Detected')
} else {
console.log('Click Detected')
}
}
window.addEventListener('mousedown', downListener)
window.addEventListener('mousemove', moveListener)
window.addEventListener('mouseup', upListener)
There are three new variables:
- threshold – the minimum amount of pixels the mouse needs to be moved to become a drag
- startingX – the initial X position of the mouse on mousedown
- startingY – the initial Y position of the mouse on mousedown
We modified two of our event listener functions:
- mousedown – now also resets the startingX and startingY variables to the initial mouse position
- mousemove – now only sets the drag boolean if the mouse moved the threshold amount in pixels in any direction.
View the JSFiddle with the included drag threshold code here.
There is, of course, various syntax you can use to achieve the same effect, along with tons of different helper libraries. The code above should work as a vanilla JS solution for most use cases.
If you found this guide helpful, check out more JavaScript coding guides in our JavaScript Section.