How to Create Global Events in Vue 3 π
There no more internal event bus in Vue 3. But don’t fret, we have a workaround for how to create global events in Vue 3.
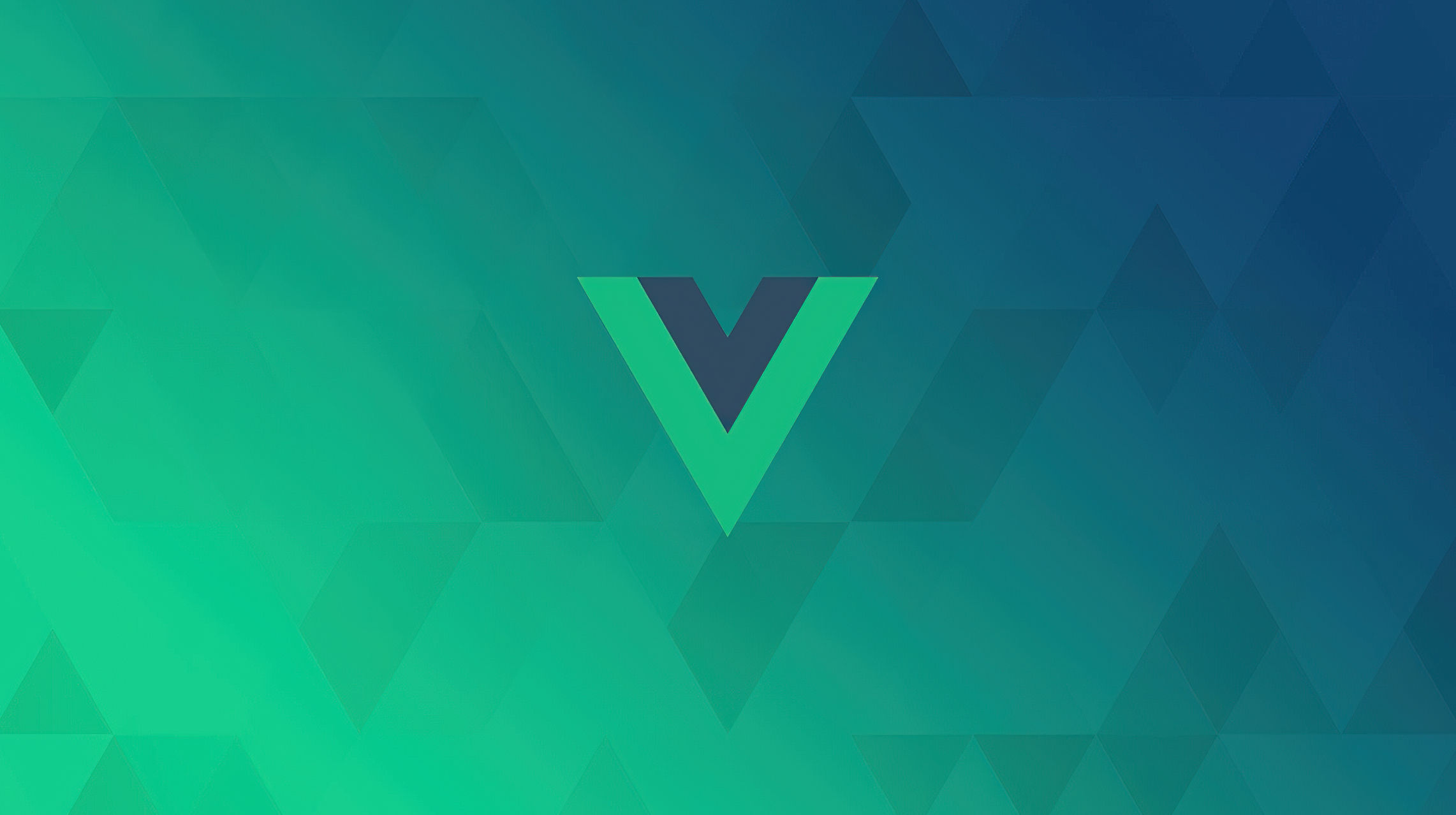
Many Vue 2 coders have become proficient in using its built-in event bus, only to discover that itβs been removed in Vue 3. Luckily if using global events via an event bus is your thing, there is a workaround. Here is how to create global events in Vue 3 using an event bus.
How to Create Global Events in Vue 3 (Solution) π
Global Events, also known as an Event Bus, can be created using a 3rd party emitter interface such as Mitt. Letβs look at how we can implement it into a project.
The Mitt package weighs in at less than 200 bytes. Making is a very tiny additional payload onto your project.
First, install the Mitt package:
npm install mitt
Next, inject the global emitter inside your main.js
file:
// main.js
import { createApp } from 'vue'
import App from './App.vue'
import mitt from 'mitt'
const emitter = mitt()
const app = createApp(App)
app.config.globalProperties.emitter = emitter
app.mount('#app')
Setup a component to fire an event:
// Emitting Component File
export default {
mounted() {
this.emitter.emit('techozu', 'Component Mounted!')
}
}
In this example, we emit when a component is mounted, but you can emit from anywhere in your code, such as a button click.
Setup a component to listen to and react to an event:
//Receiving Component File
export default {
mounted() {
this.emitter.on('techozu', value => console.log('RECEIVED!', value))
},
beforeDestroy() {
this.emitter.all.clear()
}
}
With the code above, the small Mitt package is made available to any component in your entire application.
If you have components that have event listeners in them, which are created and destroyed periodically, make sure to destroy the listeners when the component is destroyed by using this.emitter.off('eventID')
or this.emitter.all.clear()
.
You can fire an event from any component in your project and listen to the event in any other component. This gives you the freedom to interconnect your hierarchy and not to emit events solely between descendants.
Here are some additional Mitt functions that may be useful:
this.emitter.all.clear() // Clear all events
this.emitter.off('eventID') // Clear event by ID
this.emitter.off('eventID', handler) // Clear specific handler by eventID
this.emitter.on('*', handler) // Listen to all events
When to use an Event Bus in Vue 3?
An event bus can be used anytime you are trying to pass data between two or more components, specifically if the components are not direct descendants of one another. An event bus can also be used as a direct replacement for Vues child/parent emit functions.
Although state control with Vuex performs much of the same functionality as an event bus, it sometimes unnecessarily complicates your code. If you find yourself setting up a Vuex store to simply pass events between components, you should consider potentially using an event bus instead.
Note that the Vue development team discourages the use of event buses because they eventually become difficult to maintain. However, for very small projects, they can be a solid solution.
We hope you found this guide helpful. For more Vue 3 tips and tricks, check out our Vue 3 Coding Section.