How to Compare Arrays in Javascript
Learn multiple methods to compare two arrays in Javascript, including identical or mixed values.
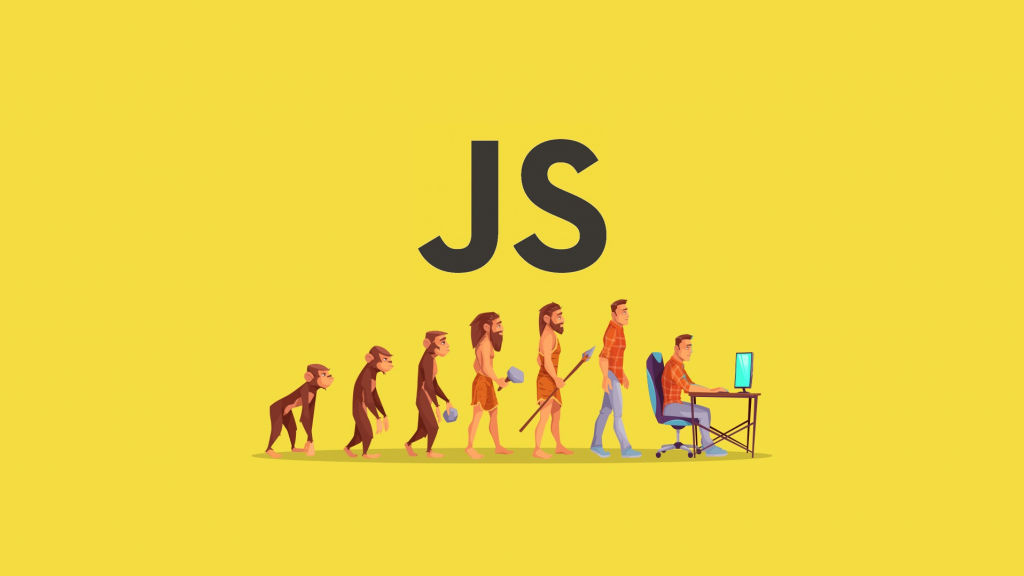
This article will cover multiple methods for comparing two arrays in Javascript. Before picking a solution, you should identify what kind of comparison you want to accomplish. Do you want to ensure that both arrays are identical with the same values in the same order? Or do you want to verify that both arrays have the same values? We will cover both scenarios and more below. Keep in mind there are many ways to accomplish this task, and it all comes down to your preferred balance between syntax simplicity and performance.
How to Compare Arrays in Javascript
The solutions listed below will cover multiple comparison scenarios, including array intersections and differences. If you know you will be dealing with huge arrays, it may be necessary to fine-tune the solutions to improve performance.
How to Check If Two Arrays Are Identical
Here is one way you can check if two arrays are identical. Meaning that both arrays have the same values in the same position.
let arrayOne = ['a','b','c','d']
let arrayTwo = ['a','b','c','d']
function isEqual(array1, array2) {
if (array1.length !== array2.length) return false;
return array1.every((value, index) => value === array2[index])
}
let result = isEqual(arrayOne, arrayTwo);
console.log(result)
//OUTPUT
//true
In this solution, we created a function to make our comparison code easily reusable. The first thing we check for is if the two arrays are of different lengths. That’s a simple and important check since there is no point in iterating the arrays if we know they are different offhand. We then use the every prototype to compare each element of both arrays. The every function runs a test on each element of the array you are using it on. In this case, we use the index to compare the values of both arrays.
Use a Library LoDash _.isEqual
If you find yourself writing a lot of functions to work with arrays and functions, you can consider using a library. LoDash is a great option and has a built-in function to check if two arrays are equal. After including the LoDash library, you can use the isEqual function.
let arrayOne = ['a','b','c','d']
let arrayTwo = ['a','b','c','d']
let result = _.isEqual(arrayOne, arrayTwo)
console.log(result)
//OUTPUT
//true
Honorable Mention – JSON.Stringify
One very simple way to compare two arrays is to turn them into strings and then compare both strings. This solution makes our code very simple but does not perform particularly well. We may also run into issues where the string representation of some values does not match, giving us errors that are difficult to debug.
let arrayOne = ['a','b','c','d']
let arrayTwo = ['a','b','c','d']
function isEqual(array1, array2) {
if (array1.length !== array2.length) return false;
return JSON.stringify(array1) === JSON.stringify(array2);
}
let result = isEqual(arrayOne, arrayTwo)
console.log(result)
//OUTPUT
//true
How to Check If Two Arrays Have the Same Values
Here is how we can check if two arrays have the same values but not necessarily in the same order.
let arrayOne = ['a','b','c','d']
let arrayTwo = ['d','b','a','c']
function isEqual(array1, array2) {
if (array1.length !== array2.length) return false;
let matching = array1.filter(val => array2.includes(val))
if (matching.length == array1.length) return true;
return false
}
let result = isEqual(arrayOne, arrayTwo);
console.log(result)
//OUTPUT
//true
Again, we first check if both arrays are the same length to save us some compute time. Next, we perform a filter operation on one array, and in it, we use the included prototype to see if the second array has each value at some index. This filter operation will return an array of matches, so if the length is the same as our input array, we know that both our compared arrays have the same values in them.
How to Find Matching Values Between Two Arrays (Intersection)
Here is how you can get the intersection of arrays. An intersection is simply a comparison of two arrays that returns the values in both arrays.
let arrayOne = ['a','b','c','d']
let arrayTwo = ['a','b','3','4']
let intersection = arrayOne.filter(val => arrayTwo.includes(val))
console.log(intersection)
//OUTPUT
//["a","b"]
How to Find Values Not In Both Array (Difference)
Here is how you can find the difference between two arrays. The difference is the inverse of the intersection. It is all the values that both the arrays do not share.
let arrayOne = ['a','b','c','d','e']
let arrayTwo = ['a','b','3','4']
let diffOne = arrayOne.filter(val => !arrayTwo.includes(val))
let diffTwo = arrayTwo.filter(val => !arrayOne.includes(val))
let difference = diffOne.concat(diffTwo)
console.log(difference)
//OUTPUT
//["c", "d", "e", "3", "4"]
There you have it. You now know how to compare arrays in Javascript.
For more helpful tutorials, please check out our Javascript coding section.