Navigator Clipboard API DOMException Javascript Fix
Learn the cause and solution for the uncaught DOMException error when working with the Clipboard API in JavaScript.
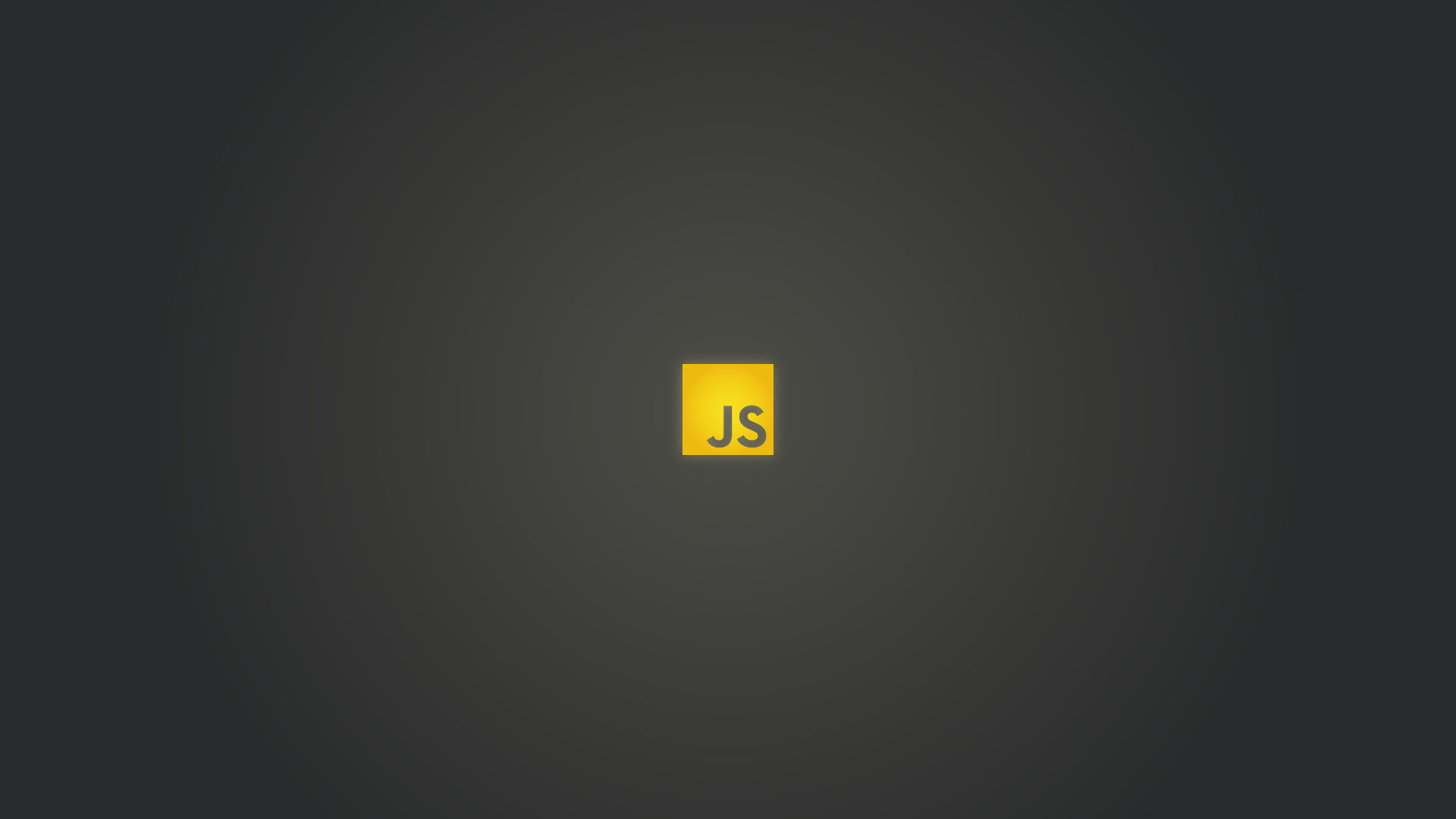
If you’re working with the Clipboard API and using the writeText() or readText() you might run into an unexpected error when testing your code. The DOMException error is odd and tough to figure out, but rest assured, we have you covered. Here is how to fix the DOMException Document is not focused error.
DOMException: Document is not focused Fix
If you are seeing Uncaught DOMException: Document is not focused when using navigator.clipboard.readText()
or navigator.clipboard.writeText()
, it is likely because you have the developer console up, and you are not currently focused on the main window.
This issue happens a lot when developers work directly out of the DevTools console while they are testing code. If this is the case for you, here is a code snippet you can use as a workaround to get the Clipboard API working when you are testing it via the developer console.
async function copyOperation(text) {
await navigator.clipboard.writeText(text)
console.log('Copy Success!')
}
setTimeout(() => { copyOperation('Hello from Techozu') }, 3000)
The code above uses the setTimeout()
function to run the navigator.clipboard
operations. Once you hit enter to execute the code above, quickly click back into the main browser window and wait for the code to run. Your Clipboard API operations will run after the set timeout delay. This delay will give you some time to refocus back into the main window.
The DOMException makes sense overall. When you are focused on executing code in the DevTools window, you are not focused on the main document. If your JavaScript is executing from the main page, make sure that your DevTools are either closed by hitting CTRL+W, or minimized or unfocused. You can arrange your browser window and DevTools window side by side and click into your browser window while executing the code. This way, you can still see console logs but will not take focus away.
alert
windows can also cause DOMExceptions with navigator.clipboard
You may also run into errors if you are using the navigator.clipboard functions and alerts to debug your code. An alert window will take focus away from the main page and which can cause the “Document is not focused” error. Let’s take a look at a code example that could cause issues.
//This code can cause issues
let text = 'Hello from Techozu'
navigator.clipboard.writeText(text);
alert("Copied the text: " + text);
Clipboard API functions return promises, so to fix the code above, you either need to change the alert to a
console.log
or await the navigator.clipboard response. Let’s look at an example.
let text = 'Hello from Techozu'
navigator.clipboard.writeText(text).then(() => {
alert("Copied the text: " + text);
})
The circumstances needed to generate the DOM error are a bit unorthodox. But hopefully, you now better understand what is causing the DOMException error when using the Clipboard API and how to fix it.
We hope the solutions in this guide worked for you. Check out some of our other JavaScript guides, tips, and tricks in our JavaScript Coding Section.