How to Check if Vue is in Development Mode?
Learn how to check if Vue is in Development mode and separate any development and production logic.
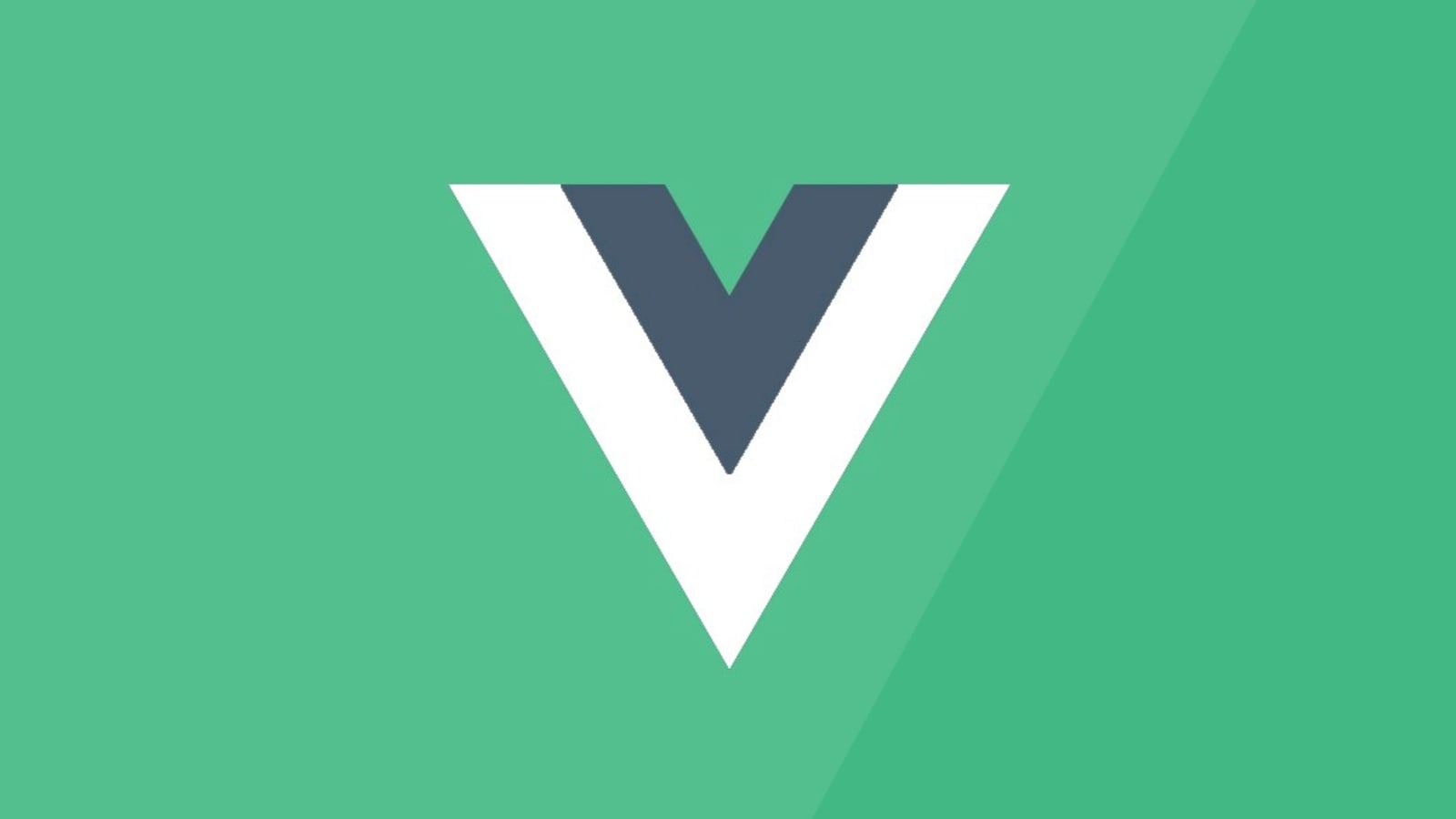
While development and production environments should be nearly interchangeable, writing development-specific code or setting development-specific variables is sometimes necessary. This is especially true when working with APIs. When you build your code, you may have a local API URL for development and a production API URL. In this case, you will need to check your Vue app’s state. Here is how to check if Vue is in Development Mode.
How to Check if Vue is in Development Mode? (Solution)
To check if Vue is in development mode, you can use the process.env.NODE_ENV
variable.
Let’s look at a simple code example that sets a variable when a component is mounted based on whether or not you are in development or production mode.
//App.vue
export default {
name: 'App',
mounted() {
console.log(process.env.NODE_ENV)
let appState = process.env.NODE_ENV === 'development' ? 'Im in Development Mode' : 'Im in Production Mode'
},
}
While you are running your development server using npm run serve
, the NODE_ENV value will default to development
. However, when you build your app using npm run build
, webpack will change the NODE_ENV variable to production.
It’s good practice to set environment-specific variables in the vue.config.js
file.
How to Set a Development API in VUE?
One common use case for checking if Vue is in development mode is to set a specific localhost API origin while in development and an official API origin when the code is compiled for production. A good place to do this is in your `vue.config.js file. Let’s take a look at an example.
//vue.config.js
process.env.VUE_APP_API = process.env.NODE_ENV === 'localhost'
? 'https://localhost:8000' : 'https://api.officialapi.com'
const { defineConfig } = require('@vue/cli-service')
module.exports = defineConfig({
transpileDependencies: true
})
This snippet creates another process variable for us to use without our code. We can call the variable like this.
//App.vue
import axios from 'axios'
export default {
name: 'App',
mounted() {
let response = axios.get(process.VUE_APP_API + 'somePath')
},
}
It’s important to ensure that your development and production logic are properly segmented in your code. It’s best practice to make your two environments as close and interchangeable as possible. If you find yourself returning to your development code and editing to test production logic, you likely need to set proper conditions higher up in your code hierarchy.
We hope you found this guide helpful and that it improves your development flow. Check out other Vue and Javascript guides in our Javascript coding section.