How to Base64 Encode a String in Node
Learn how to encode and decode Base64 strings easily in Node JS.
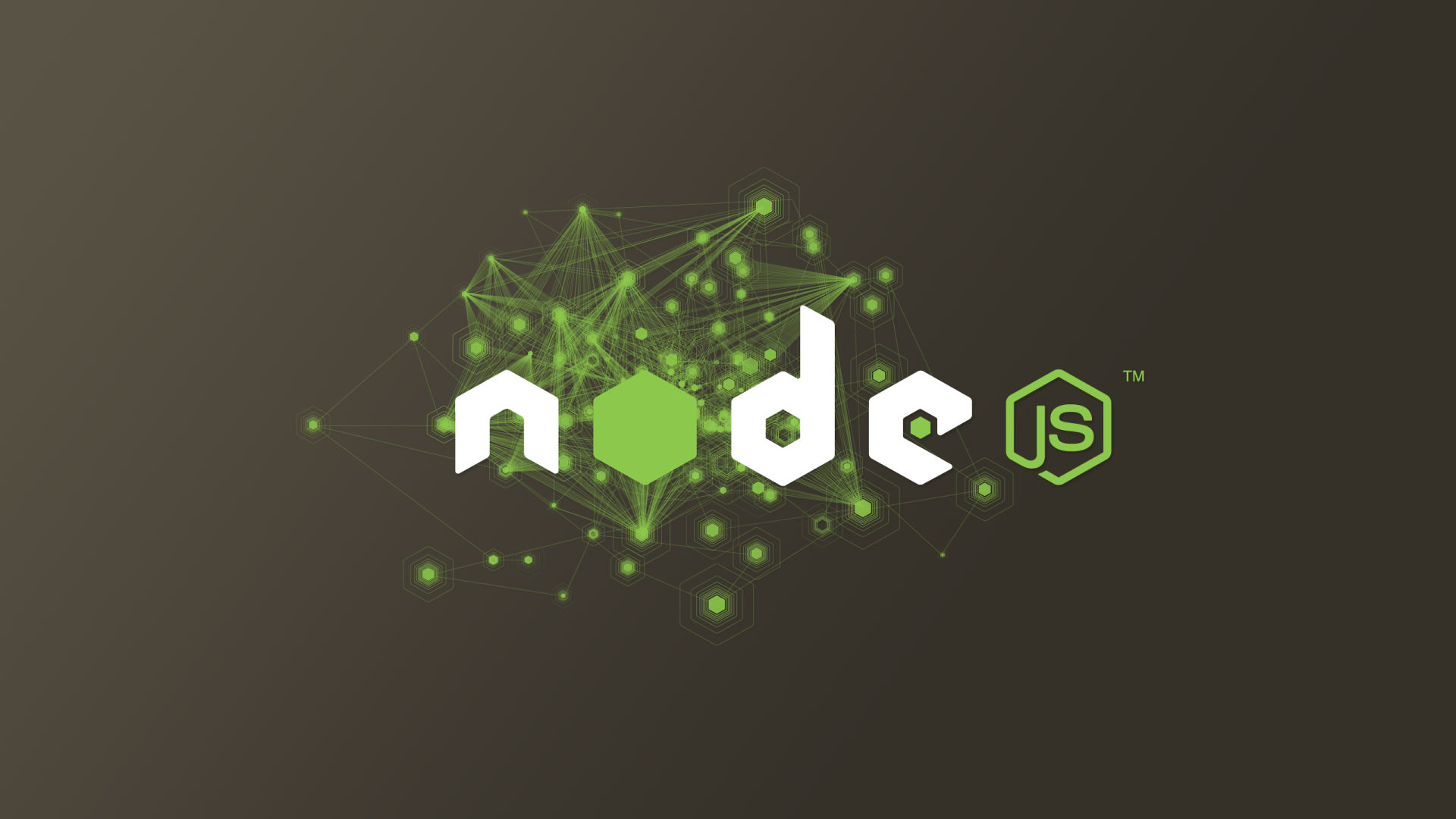
If you code for any length of time, you will eventually run into the need to either encode or decode Base64 strings. Base64 is used all over the internet and in applications to help convert binary information to content that a text interpreter could understand. Each framework and language has its own syntax for working with Base64, and Node is no exception. Here is how to Base64 encode a string in Node.
What is Base64?
Base64 is a binary-to-text encoding scheme. It is well suited for transferring data in binary formats and is very popular in web environments. Inside a website, a developer may embed an image directly into the HTML of a website by Base64 encoding the binary data of the image.
Base64 is also widely used to encode parameters in URL strings or data sent across various HTTP requests. It’s important to understand that encoding a string or any other asset into Base64 does not improve security or provide compression benefits.
How to Base64 Encode a String in Node
To Base64 encode a string in Node uses the Buffer class. The Buffer class is available to you natively inside Node without having to import any packages. To perform the encoding, we will create a buffer and then convert the Buffer to Base64. Let’s take a look at an example.
let text = 'Hello from Techozu'
let buffer = new Buffer.from(text)
let base64text = buffer.toString('base64')
console.log(text)
console.log(base64text)
//OUTPUT
//Hello from Techozu
//SGVsbG8gZnJvbSBUZWNob3p1
We use the “Buffer.from()” method to generate the Buffer we need. With the Buffer, we use the “toString()” method to convert the original string into a Base64 string we can now use. Of course, encoding the string is only half the battle. We also need to be able to decode it.
How to Base64 Decode a String in Node
To Base64 decode a string in Node, we will again use the Buffer class. The process is almost identical to encoding a string but with minor changes.
let base64text = 'SGVsbG8gZnJvbSBUZWNob3p1'
let buffer = new Buffer.from(base64text, 'base64')
let textAgain = buffer.toString('utf8')
console.log(base64text)
console.log(textAgain)
//OUTPUT
//SGVsbG8gZnJvbSBUZWNob3p1
//Hello from Techozu
To decode the string, we create a Buffer with the “Buffer.from()” method, but we pass a “base64” parameter to identify that our input is already base64 encoded. Next, we convert the Buffer to a string by using the “toString()” method with an encoding format parameter. In the coding example, we used “utf8” but you can also use “ascii” or any other encoding you may need.
Now you know how to Base64 encode and decode a string in Node. With the built-in Node classes, this process couldn’t be more straightforward.
We hope you found this guide helpful. If you would like more help, please check out our Javascript coding section.