How to Add and Remove Event Listeners in Fabric JS
Take your app to the next level by learning how to add and remove event listeners in Fabric JS.
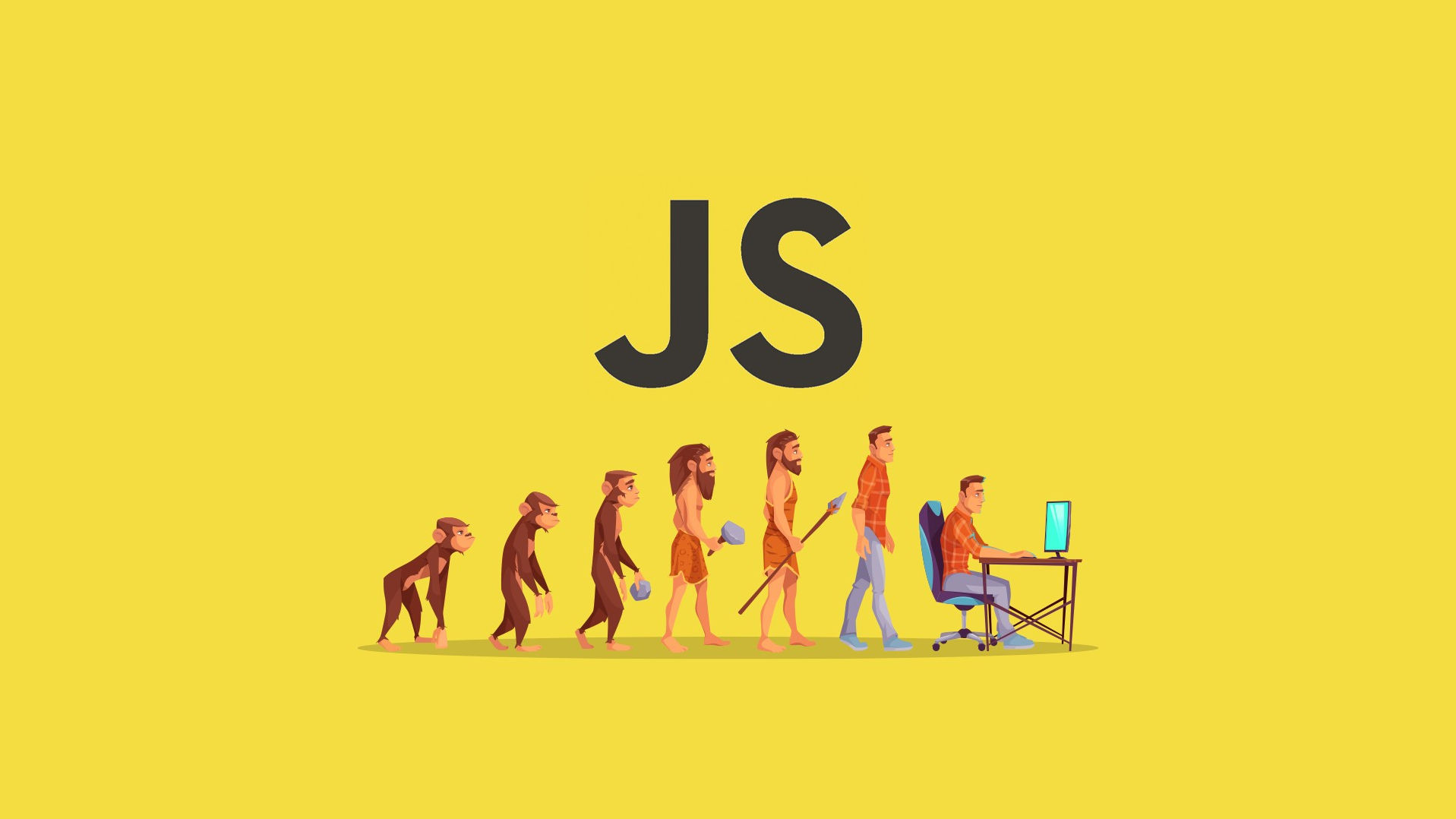
Using event listeners is standard practice when coding in Javascript. The Fabric JS library is no exception, and you will need to tie into multiple events while building up your project. Here isĀ how to add and remove event listeners in Fabric JS.
How to Add and Remove Event Listeners in Fabric JS
In Fabric JS, event listeners are added using the on()
method and removed with the off()
method. There are 26 events you can attach listeners to, plus custom events you can create.
Canvas
- after:render
- before:render
- canvas:cleared
Mouse
- mouse:over
- mouse:out
- mouse:down
- mouse:up
- mouse:move
- mouse:wheel
Object
- object:added
- object:modified
- object:moving
- object:over
- object:out
- object:removed
- object:rotating
- object:scaling
- object:selected
Selection
- before:selection:cleared
- selection:cleared
- selection:created
Text
- text:editing:entered
- text:editing:exited
- text:selection:changed
- text:changed
Path
- path:created
The adding and removing code snippets below will work for any of the events above.
Adding Event Listeners in Fabric JS
To add an event listener, use the canvases on()
method with the event as the first parameter and the event handler as the second.
let myHandler = function(e) {
console.log('Doing something with the event', e)
}
//This will listen for when an object is added
canvas.on('object:added', myHandler)
Once the event is registered, your event handler will be triggered each time the event is triggered. To make your application dynamic, you may want to toggle events on and off based on user action. Check the code snippet below for that solution.
If you are adding a Fabric JS canvas into a component-based framework like VueJS, be sure to always remove the event handlers when a component is destroyed.
Removing Event Listeners in Fabric JS
To remove an event listener, use the canvases off()
method. The first parameter will be the event, and the second parameter will be the original event handler you used to create the listener.
let myHandler = function(e) {
console.log('Doing something with the event', e)
}
//This will listen for when an object is added
canvas.on('object:added', myHandler)
//This will remove the object added event
canvas.off('object:added', myHandler)
Make sure to move your event handlers into their own functions to make it easy to remove the event listeners later.
If you would like to remove all event listeners in your Fabric JS canvas, you can use this one-liner:
canvas.__eventListeners = {};
Creating and Triggering Custom Events in Fabric JS
You can create a custom event listener by passing a custom name to the on()
method. To later fire your event, you would use the trigger()
method.
let myHandler = function(e) {
console.log('Doing something with the event', e)
}
//This will listen for when an object is added
canvas.on('techozu:hello', myHandler)
canvas.trigger('techozu:hello', 'some data')
The second parameter in the trigger method will be the options that will be passed on to your event handler.
Hopefully, you now have a firm grasp of how to add and remove event listeners in Fabric JS. Using event handlers will allow you to create a much more unique experience for people when using your application.
For more Fabric JS tips, tricks, and guides, please check out our Fabric JS section.